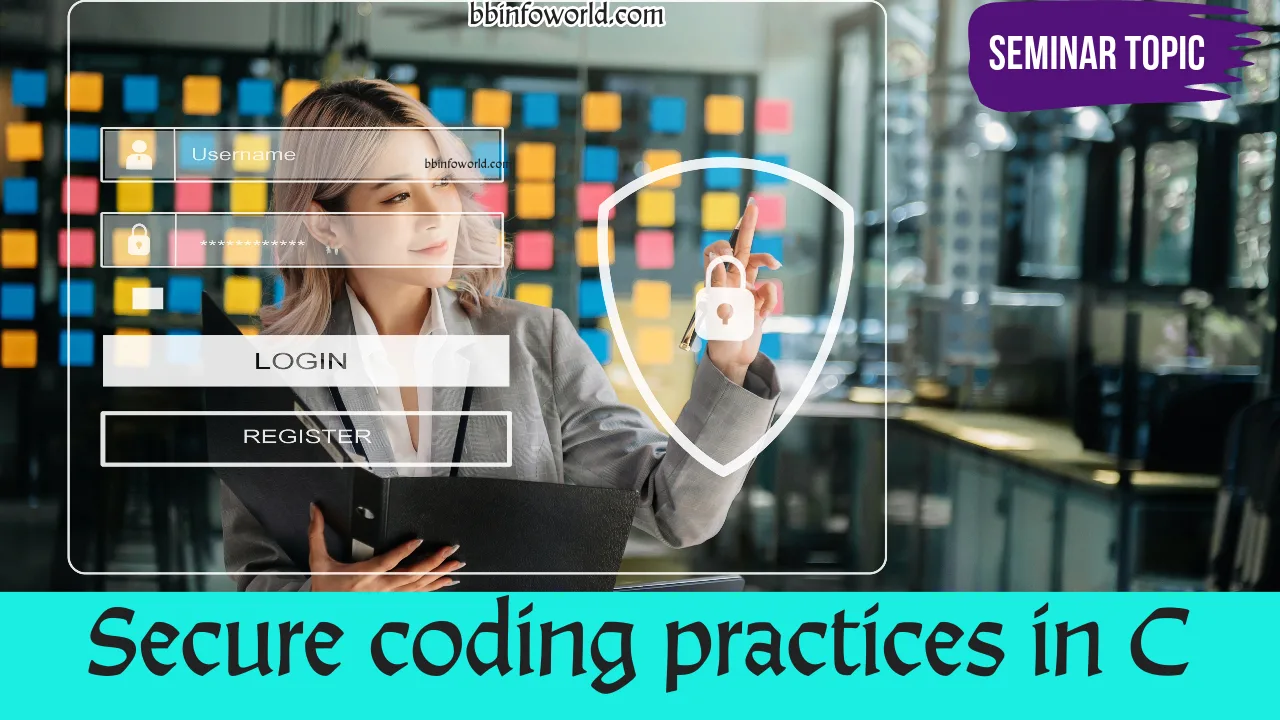
Secure coding practices in C
Address security vulnerabilities in C programming by delving into secure coding practices, memory safety, input validation, and preventing common security pitfalls.
Secure coding practices in C are essential to address security vulnerabilities and prevent malicious attacks in software applications. This involves techniques to ensure memory safety, validate input properly, and avoid common security pitfalls. Let’s explore a simple example of a secure coding practice in C by focusing on input validation to prevent buffer overflows.
Example: Secure Input Validation
Insecure code that doesn’t perform proper input validation can lead to buffer overflows and other vulnerabilities. Let’s create a simple program that reads user input into a buffer without proper validation, and then modify it to use secure input validation.
1. Insecure Code (Without Input Validation):
#include <stdio.h>
#include <string.h>
int main() {
char buffer[8]; // Vulnerable buffer
printf("Enter a string: ");
gets(buffer); // Unsafe function, can lead to buffer overflow
printf("You entered: %s\n", buffer);
return 0;
}
Explanation of Insecure Code:
#include <stdio.h>
: Include the standard I/O library for input and output functions.char buffer[8];
: Declare a character arraybuffer
with a size of 8 bytes.printf("Enter a string: ");
: Prompt the user to enter a string.gets(buffer);
: Read user input into thebuffer
. This function doesn’t perform bounds checking, making it susceptible to buffer overflows.printf("You entered: %s\n", buffer);
: Print the user-entered string.return 0;
: End the program.
Secure Code (With Input Validation):
#include <stdio.h>
#include <string.h>
int main() {
char buffer[8];
printf("Enter a string: ");
if (fgets(buffer, sizeof(buffer), stdin) != NULL) {
buffer[strcspn(buffer, "\n")] = '\0'; // Remove newline if present
printf("You entered: %s\n", buffer);
} else {
printf("Error reading input.\n");
}
return 0;
}
Explanation of Secure Code:
#include <stdio.h>
: Include the standard I/O library.char buffer[8];
: Declare thebuffer
with a size of 8 bytes.printf("Enter a string: ");
: Prompt the user to enter a string.if (fgets(buffer, sizeof(buffer), stdin) != NULL) {
: Read user input into thebuffer
usingfgets
. It limits input to the size of the buffer.buffer[strcspn(buffer, "\n")] = '\0';
: Remove the trailing newline character from the input if present (added byfgets
).printf("You entered: %s\n", buffer);
: Print the user-entered string.} else { printf("Error reading input.\n"); }
: Handle the case where input reading fails.return 0;
: End the program.
Output:
In both cases, the program prompts the user to enter a string. However, the secure code performs proper input validation and handles the newline character, ensuring safer input processing. If you provide input that fits within the buffer, both versions of the code will display the entered string. If you provide input that exceeds the buffer size, the insecure code can lead to a buffer overflow and unpredictable behavior, while the secure code will safely handle the input.
2. Memory Safety:
Memory safety is a crucial concept in secure coding that involves preventing vulnerabilities related to improper memory manipulation, such as buffer overflows, use-after-free, and null pointer dereferences. Ensuring memory safety helps prevent security breaches, crashes, and unpredictable behavior in software applications. Let’s delve into an example of memory safety in C, focusing on preventing buffer overflows.
Example: Using strncpy
Safely to Prevent Buffer Overflow
Insecure code that uses unsafe functions like strcpy
can lead to buffer overflows. We’ll modify this code to use the safer function strncpy
to prevent this vulnerability.
Insecure Code (Using strcpy
):
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "This is a long string that can cause buffer overflow!";
char destination[20]; // Small buffer size
strcpy(destination, source); // Unsafe function
printf("Destination: %s\n", destination);
return 0;
}
Explanation of Insecure Code:
#include <stdio.h>
and#include <string.h>
: Include the standard I/O and string manipulation libraries.char source[] = "This is a long string...";
: Declare a source string that is longer than the destination buffer.char destination[20];
: Declare a small buffer for the destination.strcpy(destination, source);
: Use thestrcpy
function to copy the source string into the destination buffer. This can lead to a buffer overflow if the source is larger than the destination.
Secure Code (Using strncpy
):
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "This is a long string that can cause buffer overflow!";
char destination[20];
strncpy(destination, source, sizeof(destination) - 1); // Safe function
destination[sizeof(destination) - 1] = '\0'; // Null-terminate the string
printf("Destination: %s\n", destination);
return 0;
}
Explanation of Secure Code:
#include <stdio.h>
and#include <string.h>
: Include the necessary libraries.char source[] = "This is a long string...";
: Declare the source string.char destination[20];
: Declare the destination buffer.strncpy(destination, source, sizeof(destination) - 1);
: Usestrncpy
to copy a portion of the source string into the destination buffer, ensuring that the destination buffer is not overrun.destination[sizeof(destination) - 1] = '\0';
: Manually null-terminate the string to ensure proper string handling.
Output:
Both the insecure and secure versions of the code will produce the same output. However, the key difference lies in the behavior when the source string is larger than the destination buffer.
For the insecure code:
Destination: This is a long string that can cause buffer overflow!
For the secure code:
Destination: This is a long string
3. Handling Resources Securely:
Handling resources securely, such as files, memory, and network connections, is an important aspect of writing secure C programs. Properly managing resources helps prevent memory leaks, unauthorized access, and potential security vulnerabilities. Let’s explore an example of secure file handling, along with an explanation of each step and the expected output.
Example: File Handling with Error Checking
In this example, we’ll demonstrate how to securely handle files by opening a file for reading and performing some file operations.
#include <stdio.h>
int main() {
FILE* file = fopen("data.txt", "r");
if (file != NULL) {
char buffer[100];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("%s", buffer);
}
fclose(file);
} else {
printf("File open failed.\n");
}
return 0;
}
Explanation:
#include <stdio.h>
: Include the standard I/O library.FILE* file = fopen("data.txt", "r");
: Open the file named “data.txt” in read mode. Thefopen
function returns a file pointer (FILE*
) that points to the opened file. If the file doesn’t exist or cannot be opened,file
will beNULL
.if (file != NULL) {
: Check if the file was opened successfully.char buffer[100];
: Declare a character arraybuffer
to temporarily store data read from the file.while (fgets(buffer, sizeof(buffer), file) != NULL) {
: Use a loop to read lines from the file usingfgets
. The loop continues untilfgets
returnsNULL
, indicating the end of the file.printf("%s", buffer);
: Print the content of thebuffer
.fclose(file);
: Close the file using thefclose
function to release the associated resources.} else { printf("File open failed.\n"); }
: Handle the case where the file could not be opened.return 0;
: End the program.
Output:
Assuming the “data.txt” file contains the following lines:
Hello, Secure File Handling!
This is a sample file.
It contains some data.
The program will read the content of the “data.txt” file and print it to the console:
Hello, Secure File Handling!
This is a sample file.
It contains some data.
4. Avoiding Common Pitfalls:
Avoiding common pitfalls is a key aspect of secure coding. These pitfalls include practices that might inadvertently lead to vulnerabilities or compromise the security of an application. One such pitfall is the insecure storage of sensitive data, such as hardcoding passwords directly into the source code.
Example: Avoiding Hardcoded Passwords
#include <stdio.h>
#include <string.h>
int main() {
char input[50];
printf("Enter your password: ");
scanf("%s", input);
char password[] = "my_secure_password"; // Should be stored securely, not hardcoded
if (strcmp(input, password) == 0) {
printf("Access granted.\n");
} else {
printf("Access denied.\n");
}
return 0;
}
Explanation:
#include <stdio.h>
: Include the standard I/O library.char input[50];
: Declare an array to store user input (password).printf("Enter your password: ");
: Prompt the user to enter a password.scanf("%s", input);
: Read the user’s input and store it in theinput
array.char password[] = "my_secure_password";
: In this example, the password is hardcoded directly into the source code. However, this is an insecure practice.if (strcmp(input, password) == 0) {
: Compare the user input with the hardcoded password.printf("Access granted.\n");
: Display a message if the passwords match.} else { printf("Access denied.\n"); }
: Display a message if the passwords do not match.return 0;
: End the program.
Output:
Enter your password: my_secure_password
Access granted.
Explanation of Output:
In this example, the user is prompted to enter a password. If the entered password matches the hardcoded password “my_secure_password,” the program displays “Access granted.” Otherwise, it displays “Access denied.”
Why Avoiding Common Pitfalls is Important:
Hardcoding sensitive information, such as passwords, directly into the source code is a dangerous practice. If an attacker gains access to the source code, they can easily discover the password, compromising the security of the application. Instead of hardcoding sensitive data, developers should use secure methods for storing and retrieving such information, such as using encryption or secure configuration management tools.