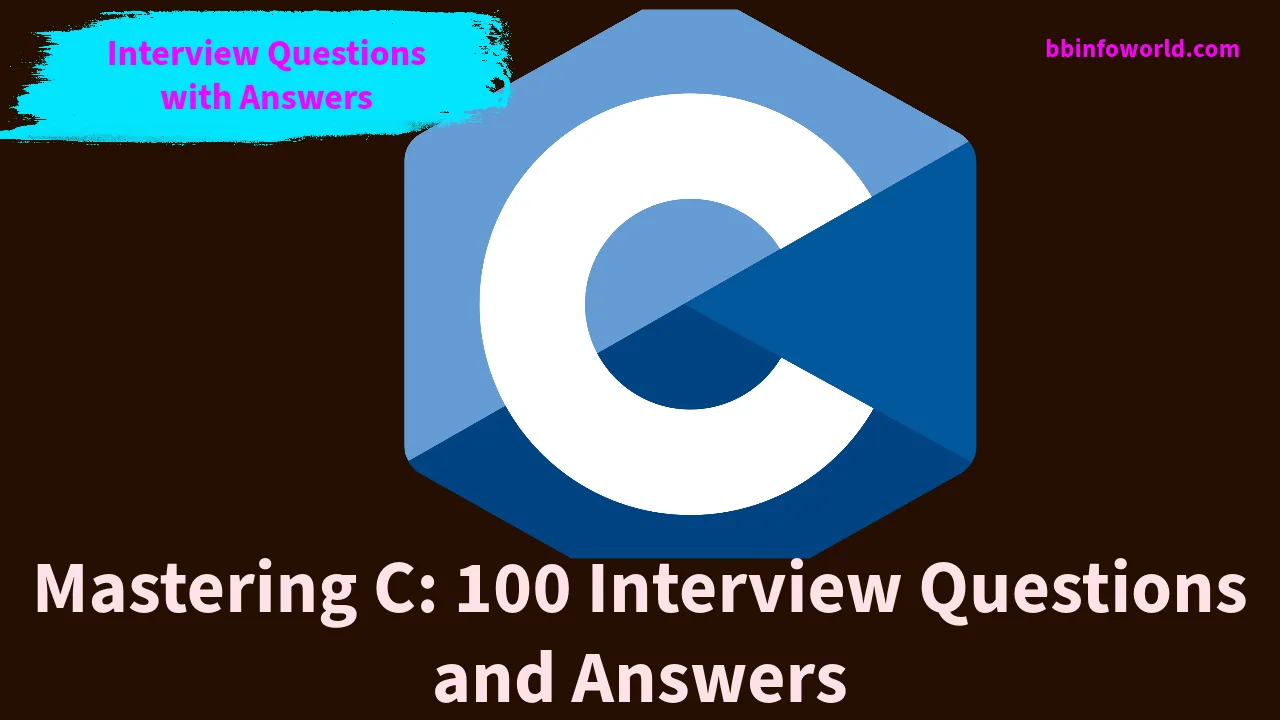
Mastering C: 100 Interview Questions and Answers
Mastering C: 100 Interview Questions and Answers
Basic C Questions:
- What is C?
- Answer: C is a general-purpose programming language developed at Bell Labs in 1972 by Dennis Ritchie. It is known for its simplicity and efficiency.
- What is the difference between C and C++?
- Answer: C is a procedural programming language, while C++ is an extension of C that supports object-oriented programming.
- What are the basic data types in C?
- Answer: Basic data types in C include
int
,char
,float
,double
, andvoid
.
- Answer: Basic data types in C include
- Explain the
sizeof
operator in C.- Answer: The
sizeof
operator is used to determine the size in bytes of a data type or variable.
- Answer: The
- What is a header file in C, and how is it used?
- Answer: A header file contains declarations for functions, variables, and constants. It is included in C programs using
#include
.
- Answer: A header file contains declarations for functions, variables, and constants. It is included in C programs using
Variables and Data Types:
- How do you declare a variable in C?
- Answer: Declare a variable by specifying its type and name, e.g.,
int age;
.
- Answer: Declare a variable by specifying its type and name, e.g.,
- What is the scope of a variable in C?
- Answer: The scope of a variable determines where it can be accessed. Variables can have global or local scope.
- Explain the difference between
int
andfloat
data types.- Answer:
int
is for integer values, whilefloat
is for floating-point (decimal) values.
- Answer:
- What is the difference between
char
andint
in C?- Answer:
char
is used for character data, whileint
is used for integer data.
- Answer:
- What is a constant in C, and how is it defined?
- Answer: A constant is a value that doesn’t change during program execution. It is defined using the
const
keyword.
- Answer: A constant is a value that doesn’t change during program execution. It is defined using the
Operators and Expressions:
- What is the assignment operator in C?
- Answer: The assignment operator (
=
) is used to assign a value to a variable.
- Answer: The assignment operator (
- Explain the concept of operator precedence in C.
- Answer: Operator precedence determines the order in which operators are evaluated in an expression. For example, multiplication (*) has higher precedence than addition (+).
- What is the ternary conditional operator (
?:
) in C?- Answer: It’s a shorthand for an
if-else
statement, used for simple conditional assignments.
- Answer: It’s a shorthand for an
- Explain the difference between pre-increment and post-increment operators (
++i
andi++
).- Answer:
++i
is pre-increment (increments and then uses the value), whilei++
is post-increment (uses the value and then increments).
- Answer:
- What is type casting in C, and how is it done?
- Answer: Type casting is the explicit conversion of one data type to another using type-specific operators like
(int)
or(float)
.
- Answer: Type casting is the explicit conversion of one data type to another using type-specific operators like
Control Flow:
- What is an
if
statement, and how is it used in C?- Answer: An
if
statement is used for conditional execution. It executes a block of code if a specified condition is true.
- Answer: An
- What is a
for
loop in C?- Answer: A
for
loop is used for iterating over a block of code a specific number of times.
- Answer: A
- Explain the purpose of the
switch
statement in C.- Answer:
switch
is used to select one of many code blocks to be executed based on the value of an expression.
- Answer:
- What is the difference between
while
anddo-while
loops in C?- Answer:
while
checks the condition before executing the loop, whiledo-while
checks it after, ensuring the loop runs at least once.
- Answer:
- What is the purpose of the
break
andcontinue
statements in C?- Answer:
break
is used to exit a loop prematurely, andcontinue
is used to skip the rest of the current iteration and continue with the next one.
- Answer:
Functions:
- How do you declare and define a function in C?
- Answer: Declare the function’s prototype, define the function’s code, and then call it.
- What is the difference between call by value and call by reference in C?
- Answer: Call by value passes a copy of the argument to the function, while call by reference passes a reference to the original argument.
- What is function recursion in C, and how is it used?
- Answer: Function recursion is when a function calls itself. It’s used to solve problems that can be broken down into smaller, similar subproblems.
- What is a function pointer in C, and how is it declared?
- Answer: A function pointer is a variable that stores the address of a function. It is declared using the function’s signature.
- Explain the concept of variable scope in C functions.
- Answer: Variables in a function have local scope, meaning they are only accessible within that function. Global variables have broader scope.
Arrays and Pointers:
- How do you declare and initialize an array in C?
- Answer: Declare an array with a specified size and initialize it with values in curly braces
{}
.
- Answer: Declare an array with a specified size and initialize it with values in curly braces
- What is a pointer in C, and how is it used?
- Answer: A pointer is a variable that stores the memory address of another variable.
- How do you allocate memory dynamically for an array using
malloc()
in C?- Answer: Use
malloc()
to allocate memory for an array, likeint* arr = (int*)malloc(5 * sizeof(int));
.
- Answer: Use
- What is pointer arithmetic, and how is it used in C?
- Answer: Pointer arithmetic involves performing arithmetic operations on pointers to navigate and manipulate memory.
- Explain the difference between an array and a pointer in C.
- Answer: An array is a collection of elements with the same data type, while a pointer is a variable that stores a memory address.
Structures and Unions:
- What is a structure in C, and how is it defined?
- Answer: A structure is a composite data type that groups together variables of different data types. It is defined using the
struct
keyword.
- Answer: A structure is a composite data type that groups together variables of different data types. It is defined using the
- What is the purpose of a union in C, and how is it defined?
- Answer: A union is a composite data type that can store different types of data in the same memory location. It is defined using the
union
keyword.
- Answer: A union is a composite data type that can store different types of data in the same memory location. It is defined using the
- What is the difference between a structure and a union in C?
- Answer: In a structure, all members have their own memory space, while in a union, all members share the same memory space.
- How do you access members of a structure in C?
- Answer: Use the dot (
.
) operator to access members of a structure.
- Answer: Use the dot (
- What is the purpose of
typedef
in C, and how is it used?- Answer:
typedef
is used to create custom data type aliases. It improves code readability and portability.
- Answer:
File Handling:
- How do you open and close a file in C?
- Answer: Use
fopen()
to open a file for reading or writing andfclose()
to close it.
- Answer: Use
- What is the difference between text mode and binary mode when opening a file in C?
- Answer: Text mode is for reading and writing text files, while binary mode is for reading and writing binary files.
- How do you read data from a file in C?
- Answer: Use functions like
fread()
orfgets()
to read data from a file.
- Answer: Use functions like
- What is the purpose of the
fprintf()
function in C?- Answer:
fprintf()
is used to write formatted data to a file.
- Answer:
- How do you check if a file exists in C before opening it?
- Answer: You can use the
access()
function or check the return value offopen()
to determine if a file exists.
- Answer: You can use the
Memory Management:
- What is dynamic memory allocation in C, and how is it done?
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
malloc()
andfree()
.
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
- How do you allocate memory for an array dynamically using
malloc()
in C?- Answer: Use
malloc()
to allocate memory, likeint* arr = (int*)malloc(5 * sizeof(int));
.
- Answer: Use
- What is a memory leak in C, and how can it be avoided?
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
free()
.
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
- Explain the purpose of the
malloc()
andfree()
functions in C.- Answer:
malloc()
is used to allocate memory, andfree()
is used to deallocate memory.
- Answer:
- What is RAII (Resource Acquisition Is Initialization) in C?
- Answer: RAII is a C programming idiom where resource management (e.g., memory allocation) is tied to the lifetime of an object. Resources are automatically released when the object goes out of scope.
Strings and Character Handling:
- What is a string in C, and how is it represented?
- Answer: In C, a string is represented as an array of characters terminated by a null character (
'\0'
).
- Answer: In C, a string is represented as an array of characters terminated by a null character (
- How do you compare two strings in C?
- Answer: Use the
strcmp()
function to compare two strings in C. It returns 0 if the strings are equal.
- Answer: Use the
- Explain the purpose of the
strlen()
function in C.- Answer:
strlen()
is used to calculate the length of a string, excluding the null character.
- Answer:
- What is the difference between a character array and a string in C?
- Answer: A character array is a collection of characters, while a string is a character array terminated by a null character (
'\0'
).
- Answer: A character array is a collection of characters, while a string is a character array terminated by a null character (
- How do you concatenate two strings in C?
- Answer: Use the
strcat()
function to concatenate two strings in C.
- Answer: Use the
Pointers and Functions:
- What is a pointer in C, and how is it used?
- Answer: A pointer is a variable that stores the memory address of another variable.
- How do you declare a pointer in C?
- Answer: Declare a pointer by specifying the data type it points to, e.g.,
int* ptr;
.
- Answer: Declare a pointer by specifying the data type it points to, e.g.,
- What is a null pointer in C, and why is it useful?
- Answer: A null pointer points to no memory location. It is useful for indicating that a pointer doesn’t point to a valid object.
- Explain the difference between
NULL
andnullptr
in C.- Answer:
NULL
is a macro defined as0
, whilenullptr
is a C++ keyword used to represent a null pointer.
- Answer:
- What is the purpose of a function pointer in C, and how is it declared?
- Answer: A function pointer is a variable that stores the address of a function. It is declared using the function’s signature.
Structures and File Handling:
- What is a structure in C, and how is it defined?
- Answer: A structure is a composite data type that groups together variables of different data types. It is defined using the
struct
keyword.
- Answer: A structure is a composite data type that groups together variables of different data types. It is defined using the
- How do you access members of a structure in C?
- Answer: Use the dot (
.
) operator to access members of a structure.
- Answer: Use the dot (
- What is a
typedef
in C, and why is it used?- Answer:
typedef
is used to create custom data type aliases. It improves code readability and portability.
- Answer:
- How do you open and close a file in C?
- Answer: Use
fopen()
to open a file for reading or writing andfclose()
to close it.
- Answer: Use
- What is the purpose of the
fprintf()
function in C?- Answer:
fprintf()
is used to write formatted data to a file.
- Answer:
Dynamic Memory Allocation and Pointers:
- What is dynamic memory allocation in C, and how is it done?
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
malloc()
andfree()
.
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
- How do you allocate memory for an array dynamically using
malloc()
in C?- Answer: Use
malloc()
to allocate memory, likeint* arr = (int*)malloc(5 * sizeof(int));
.
- Answer: Use
- What is a memory leak in C, and how can it be avoided?
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
free()
.
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
- Explain the purpose of the
malloc()
andfree()
functions in C.- Answer:
malloc()
is used to allocate memory, andfree()
is used to deallocate memory.
- Answer:
- What is RAII (Resource Acquisition Is Initialization) in C?
- Answer: RAII is a C programming idiom where resource management (e.g., memory allocation) is tied to the lifetime of an object. Resources are automatically released when the object goes out of scope.
Strings and Character Handling:
- What is a string in C, and how is it represented?
- Answer: In C, a string is represented as an array of characters terminated by a null character (
'\0'
).
- Answer: In C, a string is represented as an array of characters terminated by a null character (
- How do you compare two strings in C?
- Answer: Use the
strcmp()
function to compare two strings in C. It returns 0 if the strings are equal.
- Answer: Use the
- Explain the purpose of the
strlen()
function in C.- Answer:
strlen()
is used to calculate the length of a string, excluding the null character.
- Answer:
- What is the difference between a character array and a string in C?
- Answer: A character array is a collection of characters, while a string is a character array terminated by a null character (
'\0'
).
- Answer: A character array is a collection of characters, while a string is a character array terminated by a null character (
- How do you concatenate two strings in C?
- Answer: Use the
strcat()
function to concatenate two strings in C.
- Answer: Use the
Pointer Arithmetic and Functions:
- What is a pointer in C, and how is it used?
- Answer: A pointer is a variable that stores the memory address of another variable.
- How do you declare a pointer in C?
- Answer: Declare a pointer by specifying the data type it points to, e.g.,
int* ptr;
.
- Answer: Declare a pointer by specifying the data type it points to, e.g.,
- What is a null pointer in C, and why is it useful?
- Answer: A null pointer points to no memory location. It is useful for indicating that a pointer doesn’t point to a valid object.
- Explain the difference between
NULL
andnullptr
in C.- Answer:
NULL
is a macro defined as0
, whilenullptr
is a C++ keyword used to represent a null pointer.
- Answer:
- What is the purpose of a function pointer in C, and how is it declared?
- Answer: A function pointer is a variable that stores the address of a function. It is declared using the function’s signature.
Arrays and Pointers:
- How do you declare and initialize an array in C?
- Answer: Declare an array with a specified size and initialize it with values in curly braces
{}
.
- Answer: Declare an array with a specified size and initialize it with values in curly braces
- What is pointer arithmetic, and how is it used in C?
- Answer: Pointer arithmetic involves performing arithmetic operations on pointers to navigate and manipulate memory.
- What is an array of pointers in C, and why is it useful?
- Answer: An array of pointers is an array where each element is a pointer. It’s useful for managing multiple strings or data structures.
- How do you pass an array to a function in C?
- Answer: You can pass an array to a function by specifying its name as the argument. The function receives a pointer to the array.
- What is a two-dimensional (2D) array in C, and how is it declared?
- Answer: A 2D array is an array of arrays. It is declared with two sets of square brackets, e.g.,
int matrix[3][3];
.
- Answer: A 2D array is an array of arrays. It is declared with two sets of square brackets, e.g.,
Structures and Unions:
- What is a structure in C, and how is it defined?
- Answer: A structure is a composite data type that groups together variables of different data types. It is defined using the
struct
keyword.
- Answer: A structure is a composite data type that groups together variables of different data types. It is defined using the
- What is the purpose of a union in C, and how is it defined?
- Answer: A union is a composite data type that can store different types of data in the same memory location. It is defined using the
union
keyword.
- Answer: A union is a composite data type that can store different types of data in the same memory location. It is defined using the
- What is the difference between a structure and a union in C?
- Answer: In a structure, all members have their own memory space, while in a union, all members share the same memory space.
- How do you access members of a structure or union in C?
- Answer: Use the dot (
.
) operator to access members of a structure and the same operator for unions.
- Answer: Use the dot (
- Explain the concept of bit fields in C structures.
- Answer: Bit fields allow you to specify the number of bits each member should occupy in a structure, conserving memory.
File Handling:
- How do you open and close a file in C?
- Answer: Use
fopen()
to open a file for reading or writing andfclose()
to close it.
- Answer: Use
- What is the difference between text mode and binary mode when opening a file in C?
- Answer: Text mode is for reading and writing text files, while binary mode is for reading and writing binary files.
- How do you read data from a file in C?
- Answer: Use functions like
fread()
orfgets()
to read data from a file.
- Answer: Use functions like
- What is the purpose of the
fprintf()
function in C?- Answer:
fprintf()
is used to write formatted data to a file.
- Answer:
- How do you check if a file exists in C before opening it?
- Answer: You can use the
access()
function or check the return value offopen()
to determine if a file exists.
- Answer: You can use the
Memory Management:
- What is dynamic memory allocation in C, and how is it done?
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
malloc()
andfree()
.
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
- How do you allocate memory dynamically for an array using
malloc()
in C?- Answer: Use
malloc()
to allocate memory, likeint* arr = (int*)malloc(5 * sizeof(int));
.
- Answer: Use
- What is a memory leak in C, and how can it be avoided?
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
free()
.
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
- Explain the purpose of the
malloc()
andfree()
functions in C.- Answer:
malloc()
is used to allocate memory, andfree()
is used to deallocate memory.
- Answer:
- What is RAII (Resource Acquisition Is Initialization) in C?
- Answer: RAII is a C programming idiom where resource management (e.g., memory allocation) is tied to the lifetime of an object. Resources are automatically released when the object goes out of scope.
Strings and Character Handling:
- What is a string in C, and how is it represented?
- Answer: In C, a string is represented as an array of characters terminated by a null character (
'\0'
).
- Answer: In C, a string is represented as an array of characters terminated by a null character (
- How do you compare two strings in C?
- Answer: Use the
strcmp()
function to compare two strings in C. It returns 0 if the strings are equal.
- Answer: Use the
- Explain the purpose of the
strlen()
function in C.- Answer:
strlen()
is used to calculate the length of a string, excluding the null character.
- Answer:
- What is the difference between a character array and a string in C?
- Answer: A character array is a collection of characters, while a string is a character array terminated by a null character (
'\0'
).
- Answer: A character array is a collection of characters, while a string is a character array terminated by a null character (
- How do you concatenate two strings in C? – Answer: Use the
strcat()
function to concatenate two strings in C.