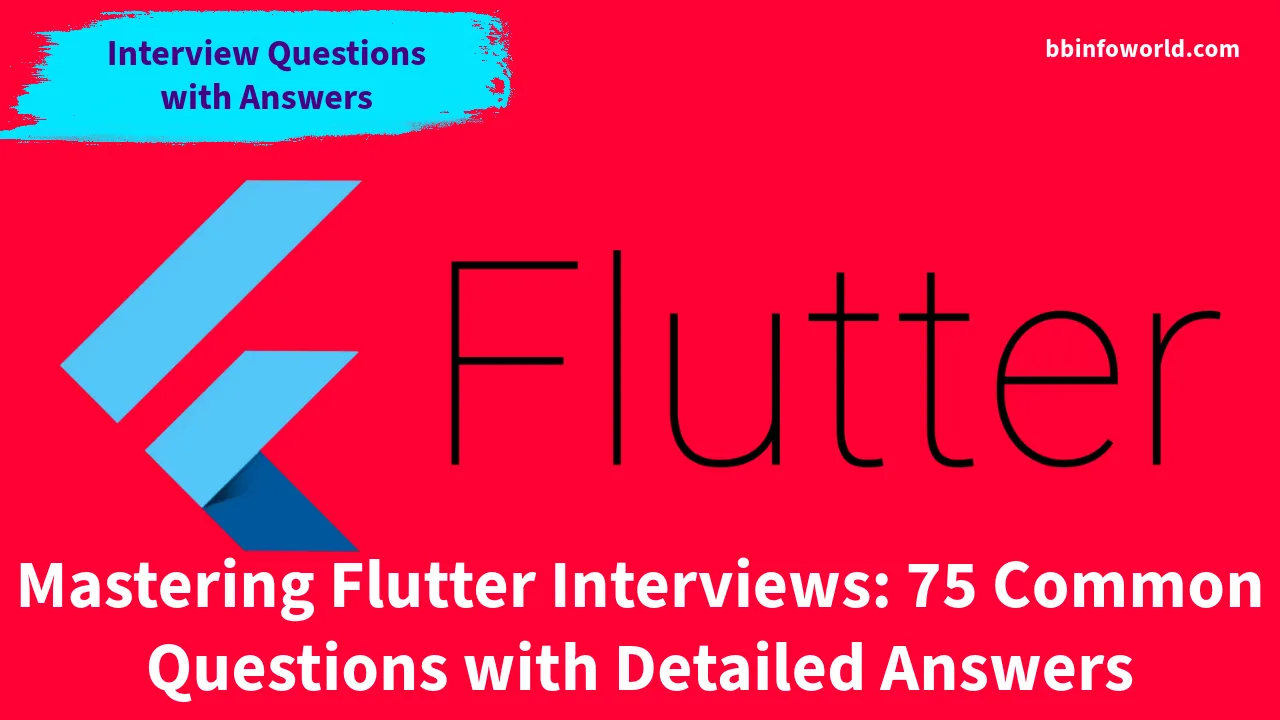
Mastering Flutter Interviews: 75 Common Questions with Detailed Answers
Mastering Flutter Interviews: 75 Common Questions with Detailed Answers
Flutter Basics:
- What is Flutter?
- Answer: Flutter is an open-source UI software development kit created by Google. It’s used for building natively compiled applications for mobile, web, and desktop from a single codebase.
- Explain the key advantages of Flutter.
- Answer: Flutter offers a rich set of advantages, including a single codebase for multiple platforms, a fast development cycle, a rich widget library, and excellent performance.
- What language is Flutter written in, and what is its primary programming language for app development?
- Answer: Flutter is written in the Dart programming language. Dart is also the primary language for developing Flutter apps.
- How does Flutter achieve native performance?
- Answer: Flutter uses a compiled programming language (Dart) and a custom rendering engine, allowing it to achieve near-native performance.
- What are widgets in Flutter?
- Answer: Widgets are the building blocks of Flutter apps. They define the user interface (UI) elements and layout of the app.
Dart Language:
- What is Dart, and why is it used in Flutter?
- Answer: Dart is a programming language used in Flutter for its fast performance, strong typing, and just-in-time (JIT) compilation.
- Explain the difference between
var
,final
, andconst
in Dart.- Answer:
var
is a dynamically typed variable,final
is an immutable variable, andconst
is a compile-time constant.
- Answer:
- What is the purpose of a
Future
in Dart, and how is it used?- Answer: A
Future
represents a potential value or error that will be available at some time in the future. It’s used for asynchronous operations in Dart.
- Answer: A
- Explain what async and await are in Dart.
- Answer:
async
is used to mark a function as asynchronous, andawait
is used to pause the function’s execution until aFuture
is complete.
- Answer:
- What is the
async*
function in Dart used for?- Answer: An
async*
function is used to create asynchronous generators, allowing the lazy generation of a sequence of values.
- Answer: An
Flutter Widgets:
- What is the difference between stateful and stateless widgets in Flutter?
- Answer: Stateless widgets are immutable and don’t change over time, while stateful widgets can change their state during their lifetime.
- What is the purpose of the
build
method in Flutter widgets?- Answer: The
build
method is responsible for returning the widget tree, defining the widget’s appearance based on its current configuration and state.
- Answer: The
- Explain the concept of a
Key
in Flutter widgets.- Answer: A
Key
is a unique identifier for a widget, used to distinguish between widgets of the same type in a widget tree.
- Answer: A
- What is the
BuildContext
in Flutter, and why is it important?- Answer:
BuildContext
represents the location of a widget in the widget tree. It’s essential for accessing resources and navigating the widget hierarchy.
- Answer:
- What is the purpose of the
setState
method in a stateful widget?- Answer:
setState
is used to trigger a rebuild of a stateful widget when its internal state changes, ensuring that the UI is updated accordingly.
- Answer:
Layout and Navigation:
- Explain the role of containers and rows/columns in Flutter layout.
- Answer: Containers are used for styling and layout, while rows/columns are used to arrange widgets in a linear fashion.
- What is the difference between
mainAxisAlignment
andcrossAxisAlignment
in Flutter?- Answer:
mainAxisAlignment
controls the alignment along the main axis (e.g., horizontal or vertical), whilecrossAxisAlignment
controls the alignment along the cross axis.
- Answer:
- What is a
ListView
in Flutter, and when is it used?- Answer: A
ListView
is a scrollable list of widgets. It’s used when you have a list of items that may not fit entirely on the screen.
- Answer: A
- Explain the purpose of the
Navigator
in Flutter.- Answer: The
Navigator
is used for managing a stack of route objects, allowing you to navigate between different screens or pages in an app.
- Answer: The
- How do you pass data between screens (routes) in Flutter?
- Answer: Data can be passed between screens using constructor arguments when navigating to a new route or using a state management solution like
Provider
orGetX
.
- Answer: Data can be passed between screens using constructor arguments when navigating to a new route or using a state management solution like
State Management:
- What is state management in Flutter, and why is it important?
- Answer: State management refers to how you manage and update the state of your app. It’s crucial for building responsive and data-driven applications.
- Explain the
setState
,InheritedWidget
, andProvider
methods of state management in Flutter.- Answer:
setState
is used for managing the state within a widget.InheritedWidget
provides a way to access data across the widget tree.Provider
is a popular state management solution for managing app-level state.
- Answer:
- What is the BLoC (Business Logic Component) pattern in Flutter?
- Answer: The BLoC pattern is a state management approach that separates the UI layer from the business logic. It uses streams and sinks to manage state changes.
- What is
GetX
, and how does it simplify state management in Flutter?- Answer:
GetX
is a lightweight state management library for Flutter that simplifies state management, dependency injection, and navigation.
- Answer:
- Explain the concept of “lifting state up” in Flutter.
- Answer: “Lifting state up” means moving the management of a piece of state to a higher level in the widget tree to share it with multiple child widgets.
User Interface (UI):
- What is a scaffold in Flutter, and why is it commonly used?
- Answer: A scaffold is a basic skeletal structure for building app screens, including an app bar, body, and bottom navigation. It’s commonly used as a starting point for UI design.
- What is a Flutter
AppBar
, and how is it used?- Answer: A
AppBar
is a material design widget used to provide top app bars in Flutter apps. It often contains titles, action buttons, and navigation.
- Answer: A
- What is a Flutter
BottomNavigationBar
, and when is it used?- Answer: A
BottomNavigationBar
provides a navigation bar at the bottom of the screen, typically used for switching between different sections or views.
- Answer: A
- How do you create a custom UI button in Flutter?
- Answer: You can create a custom button by composing other widgets, styling them, and adding gesture detectors or
InkWell
for interactivity.
- Answer: You can create a custom button by composing other widgets, styling them, and adding gesture detectors or
- What is Flutter’s
ListView.builder
, and why is it useful for long lists?- Answer:
ListView.builder
efficiently builds a scrollable list of items on-the-fly as the user scrolls, reducing memory usage for long lists.
- Answer:
Animations and Effects:
- Explain the purpose of the
AnimatedContainer
widget in Flutter.- Answer:
AnimatedContainer
animates changes to its properties like size, color, or padding, creating smooth transitions.
- Answer:
- What is a Flutter Hero widget, and how is it used for animations?
- Answer: A
Hero
widget is used for animations when transitioning elements between screens, providing a smooth visual effect.
- Answer: A
- What is the
Tween
class in Flutter, and how is it used in animations?- Answer: The
Tween
class defines a range of values used for animations. It’s used in conjunction withTweenAnimationBuilder
to create custom animations.
- Answer: The
- How do you create a custom animation in Flutter?
- Answer: You can create custom animations by using
AnimationController
,Tween
, and custom widget rebuilds or by using theRive
orFlare
libraries for more complex animations.
- Answer: You can create custom animations by using
- What is Flutter’s
AnimatedOpacity
widget, and when is it used?- Answer:
AnimatedOpacity
is used to animate the opacity (visibility) of a widget, making it appear or disappear smoothly.
- Answer:
Theming and Styling:
- How do you apply a theme to a Flutter app, and why is it beneficial?
- Answer: You can apply a theme using
ThemeData
. It helps maintain a consistent look and feel throughout the app by defining colors, fonts, and styles.
- Answer: You can apply a theme using
- What is the purpose of the
TextStyle
class in Flutter?- Answer:
TextStyle
is used to define the style of text, including font size, color, weight, and decoration.
- Answer:
- Explain the concept of ThemeData and how it affects app theming in Flutter.
- Answer:
ThemeData
is used to define the overall theme of the app, including color schemes, typography, and shape properties.
- Answer:
- How do you create custom themes in Flutter?
- Answer: Custom themes can be created by extending
ThemeData
and providing custom values for colors, fonts, and other styling properties.
- Answer: Custom themes can be created by extending
- What is Flutter’s
InkWell
widget, and how is it used for touch interactions?- Answer:
InkWell
is used to add touch ripples and custom tap effects to widgets, making them interactive and visually appealing.
- Answer:
Platform-Specific Code:
- Explain the concept of platform-specific code in Flutter.
- Answer: Platform-specific code allows you to write different code implementations for specific platforms (e.g., Android and iOS) while sharing a common codebase.
- What is Flutter’s
Platform
class, and how is it used to detect the current platform?- Answer: The
Platform
class provides information about the current platform, helping you make platform-specific decisions in your code.
- Answer: The
- How do you create platform-specific UI components or behavior in Flutter?
- Answer: You can use platform-specific plugins or conditional code to create UI components or behaviors that are specific to each platform.
- What is the purpose of the
pubspec.yaml
file in Flutter, and how does it relate to platform-specific code?- Answer: The
pubspec.yaml
file specifies dependencies and settings for your Flutter project, including platform-specific configurations.
- Answer: The
Testing and Debugging:
- What is widget testing in Flutter, and why is it useful?
- Answer: Widget testing allows you to test individual widgets in isolation, verifying their behavior and interactions.
- How do you write a unit test for a Flutter widget?
- Answer: You can write unit tests for Flutter widgets using the
test
package and thetestWidgets
function to interact with widgets.
- Answer: You can write unit tests for Flutter widgets using the
- Explain the purpose of Flutter’s DevTools, and how can it be accessed?
- Answer: DevTools is a set of debugging and performance analysis tools for Flutter. It can be accessed through a browser-based UI or integrated with Android Studio or Visual Studio Code.
- What is hot reload in Flutter, and how does it benefit the development process?
- Answer: Hot reload allows you to instantly see the effects of code changes in the running app without restarting it, speeding up the development process.
- What is Flutter’s
assert
keyword used for, and when should you use it?- Answer: The
assert
keyword is used to add assertions to your code to catch errors during development. It’s typically used for debugging and testing.
- Answer: The
- How do you debug Flutter apps using breakpoints and the Flutter debugger?
- Answer: You can set breakpoints in your code and use the Flutter debugger in IDEs like Android Studio or Visual Studio Code to step through and inspect your app’s execution.
Performance Optimization:
- What are some common techniques for optimizing the performance of a Flutter app?
- Answer: Techniques include minimizing widget rebuilds, using const constructors, optimizing images, lazy-loading data, and using the
ListView.builder
for long lists.
- Answer: Techniques include minimizing widget rebuilds, using const constructors, optimizing images, lazy-loading data, and using the
- What is Flutter’s
PerformanceOverlay
, and how does it help in performance analysis?- Answer:
PerformanceOverlay
is a widget that displays performance metrics on the screen, helping you identify areas where optimization is needed.
- Answer:
- Explain the purpose of the Flutter DevTools performance tab and how it aids in performance profiling.
- Answer: The performance tab in Flutter DevTools provides insights into frame rendering times, helping you identify performance bottlenecks.
- How do you reduce the APK size of a Flutter app?
- Answer: To reduce the APK size, you can use code splitting, remove unused resources, and optimize dependencies in your app.
- What is tree shaking in the context of Flutter, and how does it affect app size?
- Answer: Tree shaking is a process of removing unused code from the app, resulting in a smaller APK or app bundle size.
Localization and Internationalization:
- What is localization in Flutter, and why is it important for internationalization?
- Answer: Localization is the process of adapting an app’s content to different languages and regions, making it accessible to a global audience.
- How do you add localization support to a Flutter app using the
intl
package?- Answer: You can add localization support by defining message catalogs and using the
intl
package to load localized strings.
- Answer: You can add localization support by defining message catalogs and using the
- What is a
Locale
in Flutter, and how is it used for internationalization?- Answer: A
Locale
represents a specific language and region combination, allowing you to provide localized content.
- Answer: A
- Explain the purpose of the
Intl
class in Flutter’sintl
package.- Answer: The
Intl
class provides methods for formatting dates, numbers, and currencies according to the user’s locale.
- Answer: The
- How do you change the app’s locale dynamically in Flutter to support multiple languages?
- Answer: You can change the app’s locale dynamically by using the
Intl
package andLocale
objects to load the appropriate translations.
- Answer: You can change the app’s locale dynamically by using the
Package Management:
- What is the purpose of the
pubspec.yaml
file in Flutter, and how is it used to manage dependencies?- Answer: The
pubspec.yaml
file lists the dependencies for your Flutter project, and you can use thepub
command to manage and install these dependencies.
- Answer: The
- What is the
pub.dev
repository, and how is it related to Flutter package management?- Answer:
pub.dev
is the official package repository for Dart and Flutter packages. It hosts a wide range of packages that can be used in Flutter projects.
- Answer:
- How do you update dependencies in a Flutter project using the
pubspec.yaml
file?- Answer: You can update dependencies by editing the
pubspec.yaml
file to specify the desired versions, and then runningflutter pub get
to update them.
- Answer: You can update dependencies by editing the
- What is Flutter’s
pub
command, and what are some common commands used with it?- Answer: The
pub
command is used for managing Dart and Flutter packages. Common commands includeget
,upgrade
, andoutdated
.
- Answer: The
- Explain the purpose of
dependency_overrides
in thepubspec.yaml
file.- Answer:
dependency_overrides
allows you to specify different versions of a package than those defined in the dependencies section, resolving version conflicts.
- Answer:
Testing and Publishing:
- How do you write unit tests for Flutter widgets, and what is the purpose of the
testWidgets
function?- Answer: You can write unit tests for widgets using the
testWidgets
function provided by theflutter_test
package, allowing you to interact with widgets during testing.
- Answer: You can write unit tests for widgets using the
- What is Flutter’s golden file testing, and how does it work for UI testing?
- Answer: Golden file testing captures the rendered UI of a widget as an image and compares it to a reference image to detect visual regressions.
- Explain the process of running tests in a Flutter app using the
flutter test
command.- Answer: The
flutter test
command runs all the unit tests defined in your project, reporting test results and coverage.
- Answer: The
- What are Flutter driver tests, and how are they used for UI testing?
- Answer: Flutter driver tests allow you to automate UI interactions and verify the behavior of your app by running tests on a device or emulator.
- What steps are involved in publishing a Flutter app to the Google Play Store and Apple App Store?
- Answer: Steps include preparing assets and metadata, building release versions of the app, signing the app, and submitting it to the respective app stores.
Integration and APIs:
- What is Flutter’s platform channel, and how is it used to integrate native code?
- Answer: The platform channel allows Flutter to communicate with native code and access platform-specific features and APIs.
- How do you use platform-specific plugins in Flutter to access device features?
- Answer: You can use platform-specific plugins like
camera
,location
, andsensors
to access device features and APIs.
- Answer: You can use platform-specific plugins like
- What is Flutter’s
http
package, and how is it used for making HTTP requests?- Answer: The
http
package provides functions for making HTTP requests, allowing Flutter apps to interact with web services and APIs.
- Answer: The
- How do you handle device permissions (e.g., camera or location) in a Flutter app?
- Answer: Permissions can be handled using the
permission_handler
package, which allows you to request and check permissions at runtime.
- Answer: Permissions can be handled using the
- Explain the concept of asynchronous programming in Flutter, and why is it important for network requests and APIs?
- Answer: Asynchronous programming allows Flutter apps to perform non-blocking network requests and API calls, preventing UI freezes.
These 75 Flutter interview questions and answers cover a wide range of topics, from basic Flutter concepts to advanced topics like state management, animations, testing, and integration with native code and APIs. Depending on the specific job role and company, you may encounter questions that focus on particular aspects of Flutter development.