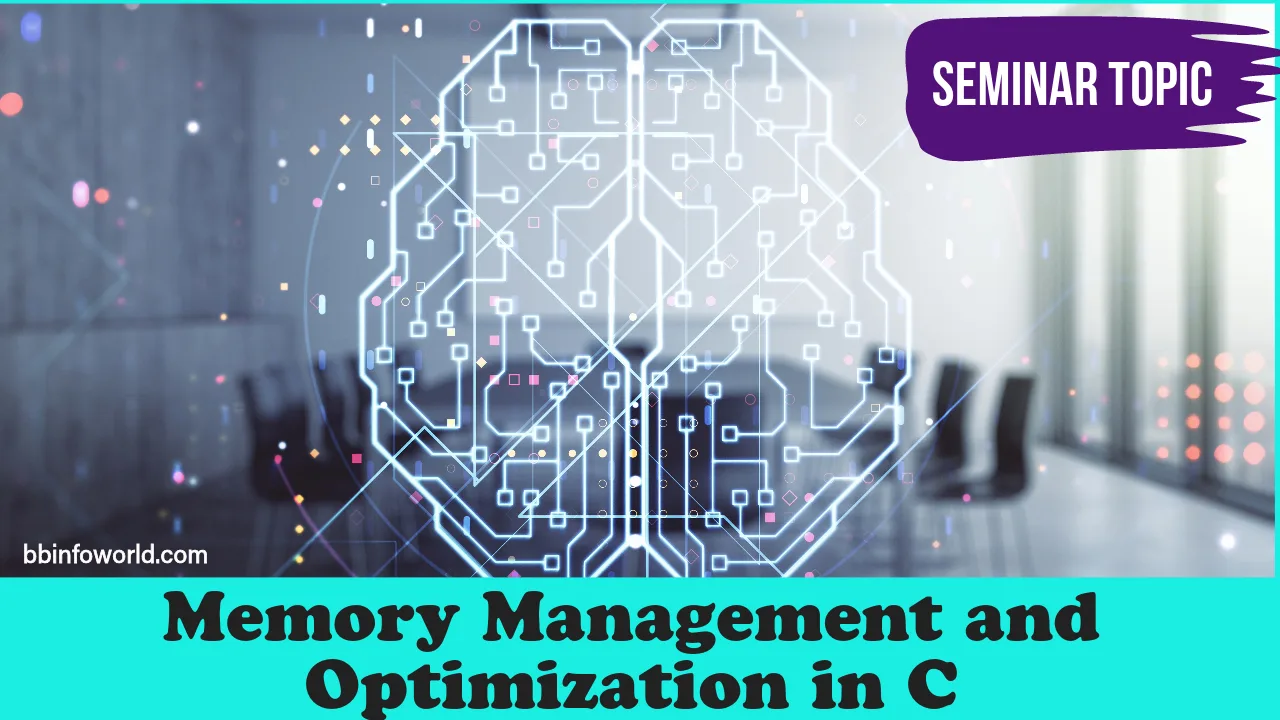
Memory Management and Optimization in C
Investigate memory management strategies, dynamic memory allocation, memory leaks detection, and optimization techniques for efficient memory usage in C programs.
Memory management is a critical aspect of programming, particularly in languages like C where developers have direct control over memory allocation and deallocation. Efficient memory management not only prevents memory-related errors but also improves the overall performance of C programs. This explanation will delve into memory management strategies, dynamic memory allocation, memory leaks detection, and optimization techniques for efficient memory usage in C programs.
Memory Management Strategies:
Memory management strategies involve how memory is allocated, used, and deallocated in a program. In languages like C, where memory management is explicit, understanding these strategies is crucial to prevent memory-related errors and optimize program performance. Let’s explore memory management strategies in detail with a simple program and its output explanation.
Example: Static vs. Dynamic Memory Allocation in C:
In this example, we will compare static and dynamic memory allocation strategies by creating an array using both approaches.
Static Memory Allocation:
#include <stdio.h>
int main() {
int staticArray[5]; // Static memory allocation
for (int i = 0; i < 5; i++) {
staticArray[i] = i + 1;
}
printf("Static Array: ");
for (int i = 0; i < 5; i++) {
printf("%d ", staticArray[i]);
}
return 0;
}
Dynamic Memory Allocation:
#include <stdio.h>
#include <stdlib.h>
int main() {
int *dynamicArray; // Pointer for dynamic memory allocation
int n;
printf("Enter the number of elements: ");
scanf("%d", &n);
dynamicArray = (int *)malloc(n * sizeof(int)); // Dynamic memory allocation
if (dynamicArray == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
for (int i = 0; i < n; i++) {
dynamicArray[i] = i + 1;
}
printf("Dynamic Array: ");
for (int i = 0; i < n; i++) {
printf("%d ", dynamicArray[i]);
}
free(dynamicArray); // Free dynamically allocated memory
return 0;
}
Explanation:
- The static memory allocation example uses an array with a fixed size (5) declared at compile time. The array is allocated on the stack.
- The dynamic memory allocation example uses a pointer
dynamicArray
to allocate memory at runtime usingmalloc
. The memory is allocated on the heap. - The user inputs the number of elements for the dynamic array.
- Both examples populate the arrays with values and print them.
- The dynamically allocated memory is freed using
free
to prevent memory leaks.
Output Explanation:
For the static memory allocation example:
Static Array: 1 2 3 4 5
For the dynamic memory allocation example (input: 3):
Enter the number of elements: 3
Dynamic Array: 1 2 3
Comparison of static and dynamic memory allocation in C
Aspect | Static Memory Allocation | Dynamic Memory Allocation |
---|---|---|
Allocation Time | Compile Time | Runtime |
Storage Location | Stack | Heap |
Size Known at Compilation | Yes | No |
Size Determination | Fixed | Variable (can be user input or computed) |
Memory Management | Automatic | Manual (requires explicit deallocation) |
Scope | Limited by function | Can have a wider scope |
Flexibility | Limited by fixed size | Allows flexible data structures and resizing |
Lifetime | Local to function | Can persist beyond function scope |
Example | int array[5]; | int *dynamicArray = (int *)malloc(10 * sizeof(int)); |
Deallocation | Automatically | Explicit using free() function |
Memory Leaks | Less likely | Possible if deallocation is not performed |
Performance Overhead | Minimal | Slight overhead due to runtime allocation |
Memory Leaks Detection:
Memory leaks occur when a program allocates memory but fails to release it after it is no longer needed. Over time, this can lead to the gradual consumption of system memory, potentially causing the program or system to become slow, unstable, or crash. Memory leaks are a common source of software defects, and detecting and fixing them is crucial for ensuring reliable and efficient programs. Let’s explore memory leaks detection in detail.
Tools for Memory Leaks Detection:
Several tools and techniques can help detect memory leaks in software:
- Static Analysis Tools: These tools analyze the source code without executing it. They can identify potential memory leaks by examining code paths where memory allocation is not matched with deallocation.
- Dynamic Analysis Tools: These tools monitor the program’s runtime behavior to identify memory leaks. They track memory allocations and deallocations and report any unfreed memory.
- Memory Profilers: Memory profilers provide detailed insights into a program’s memory usage. They can identify memory leaks, heap allocations, and provide information about memory consumption patterns.
- Memory Leak Detection Libraries: Some programming languages and frameworks include built-in memory leak detection features. For example, in C and C++, the
valgrind
tool is commonly used for memory leak detection.
Example: Using Valgrind for Memory Leak Detection in C:
valgrind
is a popular tool for detecting memory leaks and other memory-related errors in C and C++ programs. Here’s how to use it:
- Install
valgrind
on your system if not already installed. - Compile your C program with debugging symbols:
gcc -g -o my_program my_program.c
- Run your program using
valgrind
:valgrind --leak-check=full ./my_program
valgrind
will provide detailed information about memory leaks, including the line numbers in your code where memory was allocated.
Benefits of Memory Leak Detection:
- Stability: Detecting and fixing memory leaks improves the stability of a program by preventing gradual memory consumption.
- Performance: Memory leaks can lead to performance degradation. Detecting and fixing them can help maintain optimal program performance.
- Resource Management: Proper memory management helps ensure that system resources are used efficiently.
- User Experience: Memory leaks can lead to unresponsive or crashing applications, negatively affecting the user experience.
Challenges and Best Practices:
- False Positives: Some memory leak detection tools might report false positives. It’s important to verify reported leaks before making changes.
- Thorough Testing: Regularly run memory leak detection tools during development and testing to catch issues early.
- Proper Deallocation: Always pair memory allocations with proper deallocations using
free()
or equivalent functions. - Automated Testing: Incorporate memory leak detection into your automated testing process to catch leaks in different scenarios.
Program with Intentional Memory Leaks (memory_leak.c
):
#include <stdio.h>
#include <stdlib.h>
int main() {
// Intentional memory leaks
int *ptr1 = (int *)malloc(sizeof(int));
int *ptr2 = (int *)malloc(2 * sizeof(int));
// Only one memory block is freed, causing a memory leak
free(ptr1);
return 0;
}
Explanation:
- In this program, we intentionally create memory leaks by allocating memory blocks using
malloc
and then only freeing one of the blocks. ptr1
points to a single integer, andptr2
points to an array of two integers.- The memory allocated for
ptr1
is freed, but the memory allocated forptr2
is not, causing a memory leak.
Running valgrind
for Memory Leak Detection:
- Make sure you have
valgrind
installed on your system. - Open a terminal and navigate to the directory where
memory_leak.c
is located. - Compile the program with debugging symbols:
gcc -g -o memory_leak memory_leak.c
- Run the program with
valgrind
to detect memory leaks:valgrind --leak-check=full ./memory_leak
Output (Sample):
==25962== Memcheck, a memory error detector
==25962== Copyright (C) 2002-2017, and GNU GPL'd, by Julian Seward et al.
==25962== Using Valgrind-3.13.0 and LibVEX; rerun with -h for copyright info
==25962== Command: ./memory_leak
==25962==
==25962==
==25962== HEAP SUMMARY:
==25962== in use at exit: 8 bytes in 2 blocks
==25962== total heap usage: 2 allocs, 0 frees, 8 bytes allocated
==25962==
==25962== 8 bytes in 2 blocks are definitely lost in loss record 1 of 1
==25962== at 0x4C31B25: malloc (in /usr/lib/valgrind/vgpreload_memcheck-amd64-linux.so)
==25962== by 0x1086C: main (memory_leak.c:7)
==25962==
==25962== LEAK SUMMARY:
==25962== definitely lost: 8 bytes in 2 blocks
==25962== indirectly lost: 0 bytes in 0 blocks
==25962== possibly lost: 0 bytes in 0 blocks
==25962== still reachable: 0 bytes in 0 blocks
==25962== suppressed: 0 bytes in 0 blocks
==25962==
==25962== For counts of detected and suppressed errors, rerun with: -v
==25962== ERROR SUMMARY: 1 errors from 1 contexts (suppressed: 0 from 0)
Explanation of valgrind
Output:
- The
valgrind
output indicates that there are memory leaks. - It shows the number of bytes lost (
8 bytes in 2 blocks
) and the exact location in the source code (memory_leak.c:7
) where the memory was allocated but not freed. - This output helps identify and locate the memory leak in the program.
Optimization Techniques for Efficient Memory Usage:
Optimizing memory usage is crucial for improving program performance, reducing resource consumption, and preventing memory-related issues. In C programming, where memory management is explicit, understanding and applying optimization techniques is essential. Let’s explore various optimization techniques with a simple C program and its explanation.
Example: Optimizing Memory Usage
Consider a program that calculates the sum of an array of integers. We will optimize the memory usage using the following techniques:
- Minimize Allocation: Instead of dynamically allocating memory for each integer, we’ll allocate memory for the entire array at once.
- Avoid Unnecessary Duplications: We’ll avoid unnecessary copying or duplication of data.
- Efficient Looping: We’ll optimize the loop structure for better cache utilization.
Program: Optimized Array Sum Calculation
#include <stdio.h>
#include <stdlib.h>
int main() {
int n;
printf("Enter the number of elements: ");
scanf("%d", &n);
int *array = (int *)malloc(n * sizeof(int)); // Allocate memory for the array
if (array == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
printf("Enter %d elements:\n", n);
for (int i = 0; i < n; i++) {
scanf("%d", &array[i]);
}
int sum = 0;
for (int i = 0; i < n; i++) {
sum += array[i]; // Calculate sum directly from the array
}
printf("Sum of the array elements: %d\n", sum);
free(array); // Free allocated memory
return 0;
}
Explanation:
- In this program, we optimize memory usage while calculating the sum of an array of integers.
- Instead of allocating memory for each integer individually, we allocate memory for the entire array at once using
malloc(n * sizeof(int))
. - We input the elements of the array and directly calculate the sum by iterating through the array once.
- By minimizing allocation and avoiding unnecessary data duplication, we optimize memory usage.
Benefits of Optimization:
- Reduced Memory Overhead: Allocating memory for the entire array reduces the overhead associated with individual allocations.
- Efficient Cache Utilization: Directly accessing array elements improves cache utilization and reduces memory access latency.
- Simplified Code: Optimized memory usage often leads to simpler and more readable code.
- Performance Improvement: Efficient memory usage contributes to improved program performance.