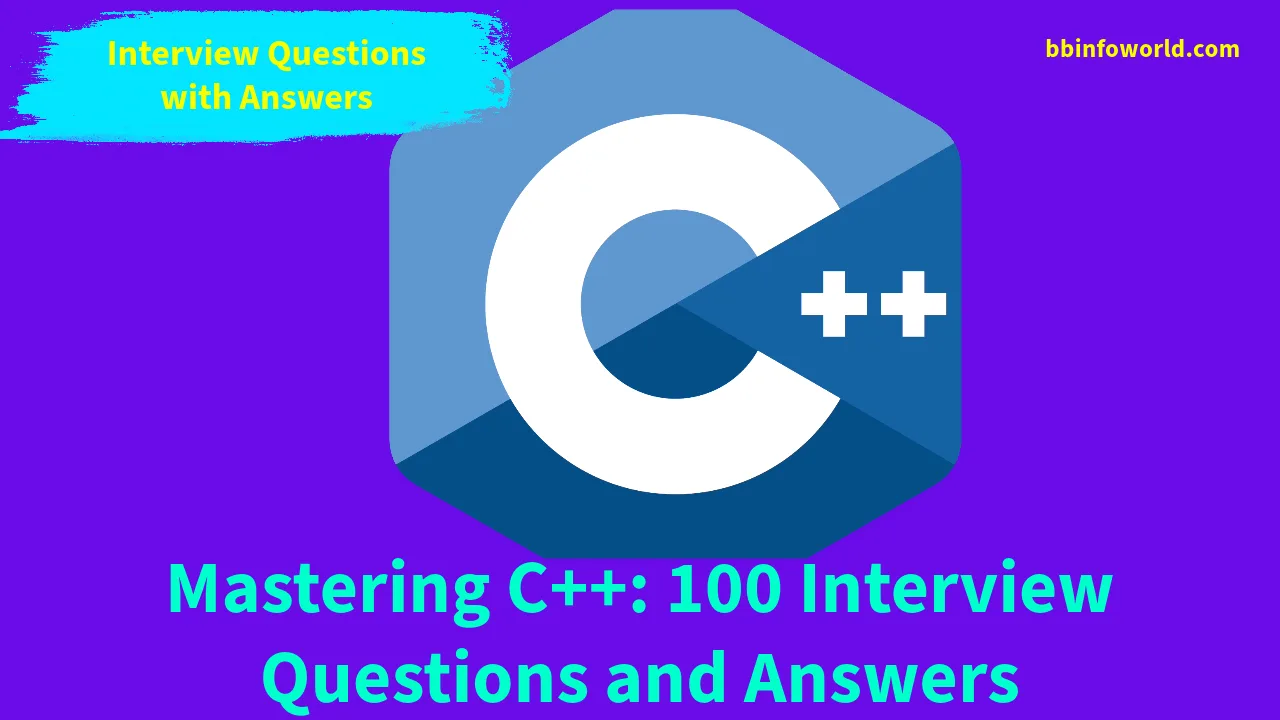
Mastering C++: 100 Interview Questions and Answers
Mastering C++: 100 Interview Questions and Answers
C++ Basics:
- What is C++?
- Answer: C++ is a high-level programming language derived from C, with features like object-oriented programming and templates.
- Explain the difference between C and C++.
- Answer: C++ adds features like classes, objects, and inheritance to C, making it an object-oriented language.
- What is the difference between
#include <iostream>
and#include "iostream"
in C++?- Answer:
<iostream>
is used for standard library headers, while"iostream"
is used for user-defined headers.
- Answer:
- What is a compiler in C++?
- Answer: A compiler translates C++ code into machine code that a computer can execute.
- What is an IDE, and name some C++ IDEs.
- Answer: An Integrated Development Environment (IDE) is a software suite for coding. Some C++ IDEs include Visual Studio, Code::Blocks, and CLion.
Variables and Data Types:
- How do you declare a variable in C++?
- Answer: You declare a variable by specifying its type and name, e.g.,
int age;
.
- Answer: You declare a variable by specifying its type and name, e.g.,
- What is the difference between
int
andfloat
data types?- Answer:
int
is for integer values, whilefloat
is for floating-point (decimal) values.
- Answer:
- What is the
sizeof
operator used for in C++?- Answer:
sizeof
returns the size in bytes of a variable or data type.
- Answer:
- Explain the purpose of the
const
keyword in C++.- Answer:
const
is used to make a variable or function parameter immutable (read-only).
- Answer:
- What are the basic data types in C++?
- Answer: Basic data types include
int
,float
,double
,char
, andbool
.
- Answer: Basic data types include
Operators and Expressions:
- What is the difference between
=
and==
in C++?- Answer:
=
is the assignment operator, while==
is the equality operator used for comparisons.
- Answer:
- Explain the concept of operator precedence in C++.
- Answer: Operator precedence determines the order in which operators are evaluated in an expression. For example, multiplication (*) has higher precedence than addition (+).
- What is the difference between pre-increment and post-increment operators (
++i
andi++
)?- Answer:
++i
is pre-increment (increments and then uses the value), whilei++
is post-increment (uses the value and then increments).
- Answer:
- What is the ternary conditional operator (
?:
) in C++?- Answer: It’s a shorthand for an
if-else
statement, used for simple conditional assignments.
- Answer: It’s a shorthand for an
- Explain the concept of type casting in C++.
- Answer: Type casting is the conversion of one data type to another, such as converting an
int
to adouble
.
- Answer: Type casting is the conversion of one data type to another, such as converting an
Control Flow:
- What is an
if
statement, and how is it used in C++?- Answer: An
if
statement is used for conditional execution. It executes a block of code if a specified condition is true.
- Answer: An
- What is a
for
loop in C++?- Answer: A
for
loop is used for iterating over a block of code a specific number of times.
- Answer: A
- Explain the purpose of the
switch
statement in C++.- Answer:
switch
is used to select one of many code blocks to be executed based on the value of an expression.
- Answer:
- What is the difference between
while
anddo-while
loops in C++?- Answer:
while
checks the condition before executing the loop, whiledo-while
checks it after, ensuring the loop runs at least once.
- Answer:
- What is the purpose of the
break
andcontinue
statements in C++?- Answer:
break
is used to exit a loop prematurely, andcontinue
is used to skip the rest of the current iteration and continue with the next one.
- Answer:
Functions:
- How do you declare and define a function in C++?
- Answer: Declare the function’s prototype, define the function’s code, and then call it.
- What is the difference between call by value and call by reference in C++?
- Answer: Call by value passes a copy of the argument to the function, while call by reference passes a reference to the original argument.
- What is function overloading in C++?
- Answer: Function overloading allows you to define multiple functions with the same name but different parameter lists.
- Explain the concept of recursion in C++.
- Answer: Recursion is a function calling itself. It’s often used to solve problems that can be broken down into smaller, similar subproblems.
- What is a default argument in a C++ function?
- Answer: A default argument is a value assigned to a function parameter that is used if the caller doesn’t provide an argument for that parameter.
Arrays and Pointers:
- How do you declare and initialize an array in C++?
- Answer: Declare an array with a specified size and initialize it with values in curly braces
{}
.
- Answer: Declare an array with a specified size and initialize it with values in curly braces
- What is a pointer in C++?
- Answer: A pointer is a variable that stores the memory address of another variable.
- How do you allocate and deallocate memory using
new
anddelete
in C++?- Answer:
new
is used to allocate memory for an object, anddelete
is used to deallocate it.
- Answer:
- Explain the difference between an array and a pointer in C++.
- Answer: An array is a collection of elements with the same data type, while a pointer is a variable that stores a memory address.
- What is pointer arithmetic, and how is it used in C++?
- Answer: Pointer arithmetic involves performing arithmetic operations on pointers to navigate and manipulate memory.
Object-Oriented Programming (OOP):
- What is a class in C++?
- Answer: A class is a blueprint for creating objects with attributes and methods.
- What is an object in C++?
- Answer: An object is an instance of a class, representing a specific entity.
- What is encapsulation in OOP, and why is it important?
- Answer: Encapsulation is the concept of bundling data and methods that operate on that data within a single unit (class). It promotes data hiding and security.
- What is inheritance in C++, and how is it implemented?
- Answer: Inheritance allows a class to inherit attributes and methods from another class. It’s implemented using the
:
operator.
- Answer: Inheritance allows a class to inherit attributes and methods from another class. It’s implemented using the
- What is polymorphism in OOP, and how is it achieved in C++?
- Answer: Polymorphism allows objects of different classes to be treated as objects of a common base class. It’s achieved through function overriding and virtual functions.
Constructors and Destructors:
- What is a constructor, and what is its purpose in C++?
- Answer: A constructor is a special member function used for initializing objects. It’s called automatically when an object is created.
- Explain the difference between a parameterized constructor and a default constructor.
- Answer: A parameterized constructor accepts arguments, while a default constructor has no arguments.
- What is a destructor in C++, and what is its purpose?
- Answer: A destructor is a special member function used to clean up resources when an object goes out of scope.
- How do you create a copy constructor in C++?
- Answer: A copy constructor is created by defining a constructor that takes an object of the same class as its parameter.
- What is the difference between a constructor and a member function in a C++ class?
- Answer: A constructor is used to initialize objects when they are created, while a member function is called to perform specific operations on objects.
Operator Overloading:
- What is operator overloading in C++, and why is it used?
- Answer: Operator overloading allows you to define custom behaviors for operators when applied to user-defined classes or types.
- Give an example of operator overloading in C++.
- Answer: Overloading the
+
operator to add two objects of a custom class.
- Answer: Overloading the
- Explain the difference between unary and binary operators in C++.
- Answer: Unary operators work on a single operand, while binary operators work on two operands.
- How do you overload the
<<
operator for output stream in C++?- Answer: Overload the
<<
operator as a friend function in your class to customize output.
- Answer: Overload the
- What is the rule of three (or rule of five) in C++?
- Answer: The rule of three (or five) states that if a class defines a destructor, copy constructor, or copy assignment operator, it should define all three (or five) to manage resources correctly.
STL (Standard Template Library):
- What is the STL in C++, and what are its main components?
- Answer: The STL is a collection of C++ template classes to provide general-purpose classes and functions with templates. Its main components include containers, algorithms, and iterators.
- What is a vector in the C++ STL?
- Answer: A vector is a dynamic array that can grow or shrink in size, providing efficient random access and insertion/removal at the end.
- Explain the difference between
std::vector
andstd::array
in C++ STL.- Answer:
std::vector
is a dynamic array with a variable size, whilestd::array
is a fixed-size array.
- Answer:
- What is an iterator in the C++ STL?
- Answer: An iterator is an object used to traverse and manipulate elements of a container, such as a vector or list.
- What is the purpose of the
std::map
container in C++ STL?- Answer:
std::map
is an associative container that stores key-value pairs in a sorted order, allowing fast lookups by key.
- Answer:
Memory Management:
- What is dynamic memory allocation in C++?
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
new
anddelete
.
- Answer: Dynamic memory allocation involves allocating and deallocating memory during program execution using functions like
- How do you allocate memory for an array dynamically using
new
in C++?- Answer: Use
new
to allocate memory, likeint* arr = new int[5];
.
- Answer: Use
- What is a memory leak in C++, and how can you avoid it?
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
delete
.
- Answer: A memory leak occurs when a program fails to deallocate memory, causing memory consumption to grow. To avoid it, always deallocate dynamically allocated memory using
- Explain the purpose of the
malloc()
andfree()
functions in C++.- Answer:
malloc()
is used to allocate memory, andfree()
is used to deallocate memory in C, whereas in C++, it’s more common to usenew
anddelete
.
- Answer:
- What is RAII (Resource Acquisition Is Initialization) in C++?
- Answer: RAII is a C++ programming idiom where resource management (e.g., memory allocation) is tied to the lifetime of an object. Resources are automatically released when the object goes out of scope.
File Handling:
- How do you open and close a file in C++?
- Answer: Use
std::ifstream
orstd::ofstream
to open a file for reading or writing, respectively, and close it withclose()
.
- Answer: Use
- What is the purpose of the
std::fstream
class in C++?- Answer:
std::fstream
is a class used for file input and output, allowing both reading and writing operations on files.
- Answer:
- How do you read data from a file in C++?
- Answer: Use
>>
orgetline()
to read data from a file, depending on the data format.
- Answer: Use
- What is the difference between text mode and binary mode when opening a file in C++?
- Answer: Text mode is for reading and writing text files, while binary mode is for reading and writing binary files. In binary mode, data is read or written as-is, without any character encoding conversions.
- How do you check if a file exists in C++ before opening it?
- Answer: You can use the
std::ifstream
constructor with the file’s name to check if it exists. If the file doesn’t exist, the stream will be in a failed state.
- Answer: You can use the
Exception Handling:
- What is exception handling in C++, and why is it used?
- Answer: Exception handling is a mechanism to handle runtime errors gracefully by using
try
,catch
, andthrow
.
- Answer: Exception handling is a mechanism to handle runtime errors gracefully by using
- Explain the use of the
try
,catch
, andthrow
keywords in C++.- Answer:
try
is used to enclose code that might raise an exception,catch
is used to handle exceptions, andthrow
is used to raise custom exceptions.
- Answer:
- What is the purpose of the
std::exception
class in C++?- Answer:
std::exception
is a base class for all standard C++ exceptions. It provides a common interface for handling exceptions.
- Answer:
- What is the difference between
throw
andthrow
with no argument in C++?- Answer:
throw
with no argument rethrows the current exception, whilethrow
with an argument throws a new exception of the specified type.
- Answer:
- What is stack unwinding in C++ exception handling?
- Answer: Stack unwinding is the process of deallocating local objects and returning control to the appropriate
catch
block when an exception is thrown.
- Answer: Stack unwinding is the process of deallocating local objects and returning control to the appropriate
Templates and Generic Programming:
- What is a template in C++?
- Answer: A template is a blueprint for creating generic classes or functions that can work with different data types.
- Explain the difference between function templates and class templates in C++.
- Answer: Function templates allow you to create generic functions, while class templates allow you to create generic classes.
- What is specialization in C++ template programming?
- Answer: Template specialization allows you to provide a specific implementation for a given data type when using a generic template.
- How do you define a function template in C++?
- Answer: Use the
template
keyword followed by the template parameter list, and then define the function as you would normally.
- Answer: Use the
- What is type inference in C++ template programming?
- Answer: Type inference allows the compiler to deduce the template argument types automatically based on the function arguments.
STL Containers:
- What is the purpose of the
std::vector
container in C++ STL?- Answer:
std::vector
is a dynamic array that can grow or shrink, providing efficient random access and dynamic sizing.
- Answer:
- What is the
std::map
container in C++ STL used for?- Answer:
std::map
is an associative container that stores key-value pairs in a sorted order for fast lookups by key.
- Answer:
- Explain the purpose of the
std::set
container in C++ STL.- Answer:
std::set
is an ordered container that stores unique elements, making it useful for maintaining a sorted collection without duplicates.
- Answer:
- What is the
std::list
container in C++ STL, and when is it used?- Answer:
std::list
is a doubly-linked list container, often used when frequent insertion and deletion of elements are required.
- Answer:
- What is the
std::deque
container in C++ STL, and how does it differ fromstd::vector
?- Answer:
std::deque
is a double-ended queue that allows efficient insertion and removal at both ends. It differs fromstd::vector
in terms of data structure and usage.
- Answer:
STL Algorithms:
- What are STL algorithms in C++, and why are they important?
- Answer: STL algorithms are a set of pre-defined functions for common operations on containers, promoting code reuse and readability.
- Explain the purpose of the
std::sort()
algorithm in C++.- Answer:
std::sort()
is used to sort elements in a container in ascending order.
- Answer:
- What is the difference between
std::find()
andstd::find_if()
in C++ STL?- Answer:
std::find()
is used to find an element with a specific value, whilestd::find_if()
is used to find an element that satisfies a given condition.
- Answer:
- What is the
std::accumulate()
algorithm in C++ used for?- Answer:
std::accumulate()
is used to compute the sum (or product) of elements in a range.
- Answer:
- Explain the purpose of the
std::transform()
algorithm in C++.- Answer:
std::transform()
applies a specified operation to each element in a range and stores the results in another container.
- Answer:
Smart Pointers:
- What are smart pointers in C++, and why are they used?
- Answer: Smart pointers are objects that manage the memory of dynamically allocated objects, helping to prevent memory leaks and manage resources.
- What is the difference between
std::shared_ptr
andstd::unique_ptr
in C++?- Answer:
std::shared_ptr
allows multiple pointers to share ownership of an object, whilestd::unique_ptr
has sole ownership.
- Answer:
- Explain the purpose of the
std::weak_ptr
in C++ smart pointers.- Answer:
std::weak_ptr
is used for non-intrusive cyclic references in cases wherestd::shared_ptr
could lead to circular references.
- Answer:
- How do you create a smart pointer in C++?
- Answer: Use
std::shared_ptr
,std::unique_ptr
, orstd::weak_ptr
withstd::make_shared()
orstd::make_unique()
for allocation.
- Answer: Use
- What is the purpose of
std::make_shared()
in C++ smart pointers?- Answer:
std::make_shared()
is a helper function that creates astd::shared_ptr
with better memory management than usingnew
directly.
- Answer:
Concurrency and Multithreading:
- What is multithreading in C++, and why is it used?
- Answer: Multithreading is a technique that allows a program to run multiple threads concurrently, improving performance and responsiveness.
- Explain the difference between a thread and a process in C++.
- Answer: A process is a separate instance of a program, while a thread is a smaller unit of a process that can run concurrently.
- What is the purpose of the
std::thread
class in C++?- Answer:
std::thread
is used to create and manage threads in C++, allowing for concurrent execution of code.
- Answer:
- How do you synchronize access to shared resources in multithreaded C++ programs?
- Answer: Synchronization can be achieved using mutexes (
std::mutex
) and other synchronization primitives like condition variables.
- Answer: Synchronization can be achieved using mutexes (
- What is a race condition, and how can it be avoided in multithreaded C++ programs?
- Answer: A race condition occurs when multiple threads access shared data concurrently, leading to unpredictable results. It can be avoided by using proper synchronization techniques like mutexes.
Advanced C++ Topics:
- What is the purpose of the
volatile
keyword in C++?- Answer:
volatile
is used to indicate that a variable may be modified by external factors and should not be optimized by the compiler.
- Answer:
- What is a lambda expression in C++, and how is it used?
- Answer: A lambda expression is an anonymous function that can capture and use local variables. It’s often used for concise callbacks and custom sorting functions.
- Explain the purpose of the C++ Standard Library (STL).
- Answer: The STL is a collection of C++ template classes and functions that provide common data structures and algorithms, promoting code reuse and portability.
- What is RAII (Resource Acquisition Is Initialization) in C++?
- Answer: RAII is a C++ programming idiom where resource management (e.g., memory allocation) is tied to the lifetime of an object. Resources are automatically released when the object goes out of scope.
- What is the purpose of the
const
keyword in C++?- Answer:
const
is used to indicate that a variable or object cannot be modified after it’s been initialized.
- Answer:
- Explain the difference between a reference and a pointer in C++.
- Answer: A reference is an alias for an existing object, while a pointer is a separate variable that stores an address.
- What is the purpose of the
inline
keyword in C++?- Answer:
inline
suggests that the compiler should perform inline expansion of a function, replacing the function call with the function code.
- Answer:
- What is the rule of three (or rule of five) in C++?
- Answer: The rule of three (or five) states that if a class defines a destructor, copy constructor, or copy assignment operator, it should define all three (or five) to manage resources correctly.
- Explain the concept of template specialization in C++.
- Answer: Template specialization allows you to provide a specific implementation for a given data type when using a generic template.
- What is the purpose of the
std::move()
function in C++? – Answer:std::move()
is used to cast an lvalue to an rvalue, allowing for efficient move semantics and avoiding unnecessary copying.