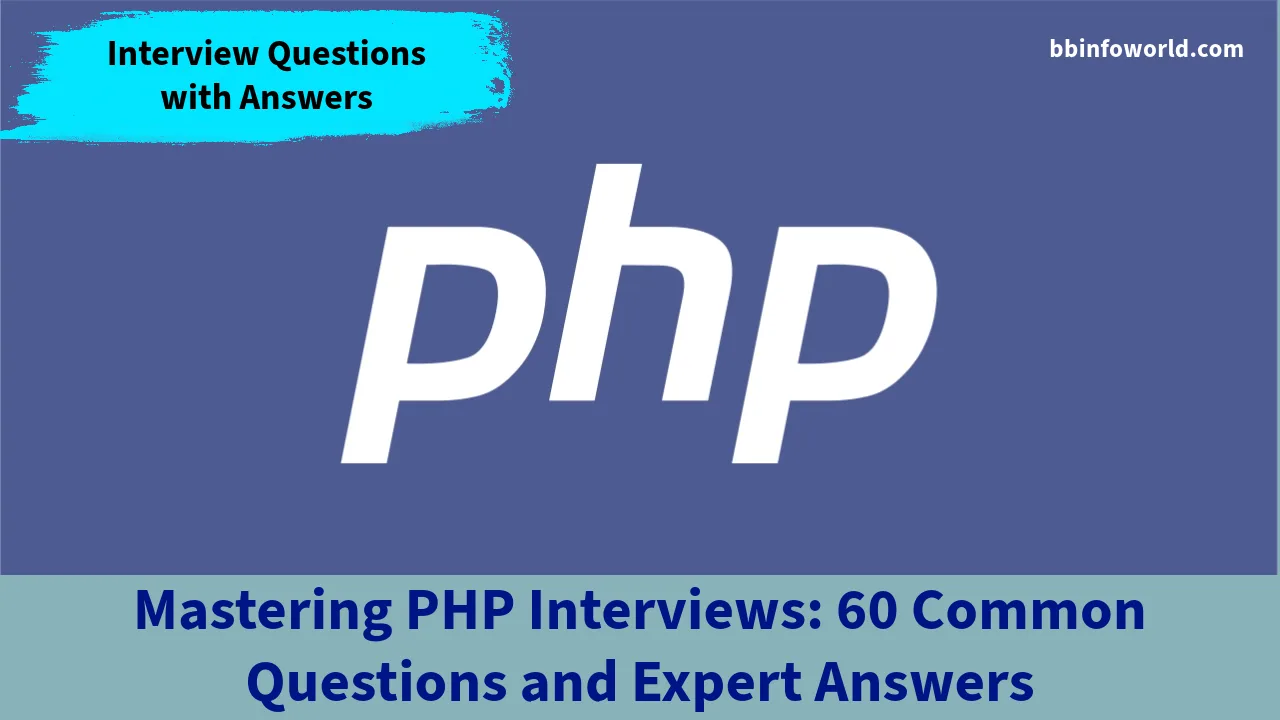
Mastering PHP Interviews: 60 Common Questions and Expert Answers
Mastering PHP Interviews: 60 Common Questions and Expert Answers
PHP Basics:
- What is PHP, and what does it stand for?
- Answer: PHP stands for “PHP: Hypertext Preprocessor.” It is a server-side scripting language for web development.
- How do you embed PHP code within HTML?
- Answer: PHP code is embedded within HTML using
<?php ... ?>
tags.
- Answer: PHP code is embedded within HTML using
- What is the purpose of PHP’s
echo
statement?- Answer: The
echo
statement is used to output data to the web browser.
- Answer: The
- Explain the difference between single quotes (
'
) and double quotes ("
) in PHP string declarations.- Answer: Single quotes are used for string literals where variables are not expanded, while double quotes allow variable interpolation.
- What are PHP variables, and how are they declared?
- Answer: PHP variables are used to store data. They are declared with a
$
sign followed by the variable name.
- Answer: PHP variables are used to store data. They are declared with a
Data Types and Operators:
- What are the basic data types in PHP?
- Answer: PHP supports data types like integer, float, string, boolean, array, object, and resource.
- Explain the difference between
==
and===
operators in PHP.- Answer:
==
checks for equality of values, while===
checks for both equality of values and data types.
- Answer:
- What is the use of the
.
operator in PHP?- Answer: The
.
operator is used for concatenating strings.
- Answer: The
- How do you declare and use constants in PHP?
- Answer: Constants are declared using the
define()
function and are accessed without the$
sign.
- Answer: Constants are declared using the
- What is type casting in PHP, and how can it be done?
- Answer: Type casting is the process of converting a variable from one data type to another. It can be done using type casting functions like
(int)
,(float)
, and(string)
.
- Answer: Type casting is the process of converting a variable from one data type to another. It can be done using type casting functions like
Control Structures:
- What are PHP control structures, and why are they used?
- Answer: Control structures are used to control the flow of a PHP program. They include
if
,else
,while
,for
, andswitch
statements.
- Answer: Control structures are used to control the flow of a PHP program. They include
- Explain the difference between
if
andswitch
statements in PHP.- Answer:
if
statements are used for conditional branching, whileswitch
statements are used for multi-branching based on a single expression.
- Answer:
- What is a PHP
foreach
loop, and how is it used to iterate over arrays?- Answer: The
foreach
loop is used to iterate over elements of an array, associative array, or object.
- Answer: The
- How can you exit a loop prematurely in PHP?
- Answer: You can use the
break
statement to exit a loop prematurely.
- Answer: You can use the
- Explain the use of the
continue
statement in PHP loops.- Answer: The
continue
statement is used to skip the rest of the current iteration and move to the next one in a loop.
- Answer: The
Functions:
- What is a PHP function, and how is it defined?
- Answer: A PHP function is a block of code that can be reused. It is defined using the
function
keyword.
- Answer: A PHP function is a block of code that can be reused. It is defined using the
- How do you pass arguments to a PHP function, and what are default arguments?
- Answer: Arguments are passed in the function declaration, and default arguments have predefined values if not provided.
- Explain the difference between
return
andecho
in PHP functions.- Answer:
return
is used to return a value from a function, whileecho
is used to output data to the browser.
- Answer:
- What is a recursive function in PHP, and why is it used?
- Answer: A recursive function is one that calls itself. It’s used for solving problems that can be broken down into smaller, similar subproblems.
- What is variable scope in PHP, and how does it work with functions?
- Answer: Variable scope defines where a variable is accessible. Variables declared within a function have local scope, while those outside have global scope.
Arrays:
- How do you declare an array in PHP, and what are the different types of arrays?
- Answer: Arrays are declared using the
array()
constructor or the[]
shorthand. PHP supports indexed arrays, associative arrays, and multidimensional arrays.
- Answer: Arrays are declared using the
- What is the difference between indexed arrays and associative arrays in PHP?
- Answer: Indexed arrays use numeric keys, while associative arrays use named keys (strings) to access values.
- How can you add elements to an array in PHP?
- Answer: You can use the
$array[] = $value
syntax to add elements to the end of an array.
- Answer: You can use the
- Explain how to remove elements from an array in PHP.
- Answer: You can use functions like
unset()
,array_pop()
,array_shift()
, orarray_splice()
to remove elements from an array.
- Answer: You can use functions like
- What is the
array_merge()
function in PHP, and how is it used to combine arrays?- Answer:
array_merge()
is used to merge two or more arrays into a single array.
- Answer:
Object-Oriented Programming (OOP):
- What is Object-Oriented Programming (OOP) in PHP, and why is it important?
- Answer: OOP is a programming paradigm that uses objects and classes to structure code. It promotes code reusability, encapsulation, and modularity.
- How do you create a class in PHP, and what are the key components of a class?
- Answer: A class is created using the
class
keyword. Key components include properties (variables) and methods (functions).
- Answer: A class is created using the
- Explain the concepts of inheritance and encapsulation in PHP OOP.
- Answer: Inheritance allows a class to inherit properties and methods from another class, while encapsulation restricts direct access to class properties, promoting data integrity.
- What is a constructor in PHP, and how is it used to initialize objects?
- Answer: A constructor is a special method called when an object is created. It’s used to initialize object properties.
- What is method overriding in PHP, and how is it achieved in child classes?
- Answer: Method overriding allows a child class to provide a specific implementation of a method inherited from a parent class. It’s achieved by declaring a method with the same name in the child class.
Exception Handling:
- What are exceptions in PHP, and why are they used for error handling?
- Answer: Exceptions are used to handle errors and exceptional conditions gracefully, allowing for more controlled error handling.
- Explain the
try
,catch
, andfinally
blocks in PHP exception handling.- Answer: The
try
block encloses code that might throw exceptions, thecatch
block handles exceptions, and thefinally
block contains code that always executes.
- Answer: The
- What is the
throw
statement in PHP, and how is it used to raise custom exceptions?- Answer: The
throw
statement is used to raise exceptions manually, allowing for custom exception handling.
- Answer: The
- What is the purpose of custom exception classes in PHP?
- Answer: Custom exception classes allow developers to define specific exception types and handling for their applications.
- How can you handle multiple exceptions in PHP using multiple
catch
blocks?- Answer: You can use multiple
catch
blocks to handle different types of exceptions individually.
- Answer: You can use multiple
File Handling:
- How do you open and close files in PHP, and what are the modes for opening files?
- Answer: Files are opened using
fopen()
and closed usingfclose()
. Modes include read (r
), write (w
), append (a
), and more.
- Answer: Files are opened using
- Explain how to read data from a file in PHP using the
fread()
andfgets()
functions.- Answer:
fread()
reads a specified number of bytes, whilefgets()
reads a line from a file.
- Answer:
- What is file uploading in PHP, and how can you handle file uploads from HTML forms?
- Answer: File uploading allows users to send files to the server. It’s handled using PHP’s
$_FILES
superglobal and themove_uploaded_file()
function.
- Answer: File uploading allows users to send files to the server. It’s handled using PHP’s
- What is file handling with directories in PHP, and how can you create, read, and delete directories and files?
- Answer: PHP provides functions like
mkdir()
,rmdir()
,opendir()
, andunlink()
to work with directories and files.
- Answer: PHP provides functions like
- What is file locking in PHP, and why is it used in multi-user environments?
- Answer: File locking prevents multiple users from accessing and modifying the same file simultaneously, ensuring data integrity.
MySQL Database Integration:
- What is MySQL, and how do you connect to a MySQL database in PHP?
- Answer: MySQL is a popular relational database management system. You can connect to it using the
mysqli
orPDO
extension in PHP.
- Answer: MySQL is a popular relational database management system. You can connect to it using the
- Explain how to perform SQL queries in PHP, including SELECT, INSERT, UPDATE, and DELETE statements.
- Answer: SQL queries are executed using functions like
mysqli_query()
orPDO
methods. SELECT retrieves data, INSERT adds records, UPDATE modifies records, and DELETE removes records.
- Answer: SQL queries are executed using functions like
- What is prepared statement in PHP MySQL, and why is it considered safer for database operations?
- Answer: Prepared statements separate SQL code from user input, reducing the risk of SQL injection attacks.
- How do you fetch data from a MySQL result set in PHP, and what are the common methods for retrieving data?
- Answer: Data is fetched using functions like
mysqli_fetch_assoc()
,mysqli_fetch_array()
, orPDO
methods.
- Answer: Data is fetched using functions like
- What is database normalization, and why is it important in database design?
- Answer: Database normalization is a process of organizing data to minimize redundancy and maintain data integrity in a relational database.
Sessions and Cookies:
- What are PHP sessions and cookies, and how are they used for user state management?
- Answer: Sessions and cookies are used to store and manage user data between requests. Sessions are stored server-side, while cookies are stored on the client-side.
- How do you start and destroy sessions in PHP?
- Answer: Sessions are started using
session_start()
and destroyed usingsession_destroy()
.
- Answer: Sessions are started using
- What is the purpose of PHP cookies, and how are they set and accessed?
- Answer: Cookies are used to store data on the client’s browser. They are set using the
setcookie()
function and accessed using$_COOKIE
.
- Answer: Cookies are used to store data on the client’s browser. They are set using the
- What is session hijacking, and how can it be prevented in PHP?
- Answer: Session hijacking is an attack where an attacker steals a user’s session. It can be prevented by using secure session management practices and storing session data securely.
- Explain the concept of session fixation in PHP and how to defend against it.
- Answer: Session fixation is an attack where an attacker sets a user’s session ID. It can be defended against by regenerating session IDs and validating session data.
Security:
- What is SQL injection, and how can you prevent it in PHP?
- Answer: SQL injection is an attack where malicious SQL queries are injected into user input. It can be prevented by using prepared statements or sanitizing user input.
- What is Cross-Site Scripting (XSS), and how can you prevent it in PHP?
- Answer: XSS is an attack where malicious scripts are injected into web pages. It can be prevented by validating and escaping user input, and by using output encoding.
- Explain the concept of Cross-Site Request Forgery (CSRF) in PHP, and how can it be mitigated?
- Answer: CSRF is an attack where an attacker tricks a user into executing actions without their consent. It can be mitigated by using anti-CSRF tokens and checking referer headers.
- What is password hashing in PHP, and why is it important for storing passwords securely?
- Answer: Password hashing is the process of converting a password into a secure hash, protecting it from exposure in case of a data breach.
- How do you implement user authentication and authorization in PHP, including user roles and permissions?
- Answer: User authentication can be implemented using sessions or tokens. Authorization involves defining roles and checking permissions for different user levels.
PHP Frameworks:
- Name some popular PHP frameworks, and explain the benefits of using frameworks for web development.
- Answer: Popular PHP frameworks include Laravel, Symfony, CodeIgniter, and Yii. Frameworks provide pre-built components, follow best practices, and speed up development.
- What is MVC architecture, and how is it implemented in PHP frameworks like Laravel?
- Answer: MVC (Model-View-Controller) is a design pattern that separates an application into three interconnected components. Laravel and other PHP frameworks use this pattern for structured development.
- Explain the concept of routing in PHP frameworks, and how are routes defined?
- Answer: Routing maps URLs to specific controller actions in PHP frameworks, defining how requests are handled.
- What is an ORM (Object-Relational Mapping) in PHP, and why is it used in web development?
- Answer: ORM is a technique used to map database tables to PHP objects, making database interactions more object-oriented and less SQL-centric.
- What are RESTful APIs, and how can you create RESTful services in PHP using frameworks?
- Answer: RESTful APIs use HTTP methods to perform CRUD operations on resources. PHP frameworks like Laravel provide tools for creating RESTful services.
These 60 PHP interview questions and answers cover a wide range of PHP topics, from the basics and data types to more advanced concepts like OOP, database integration, and security. Depending on the specific job role and company, you may encounter questions that focus on particular PHP frameworks or tools used in web development.