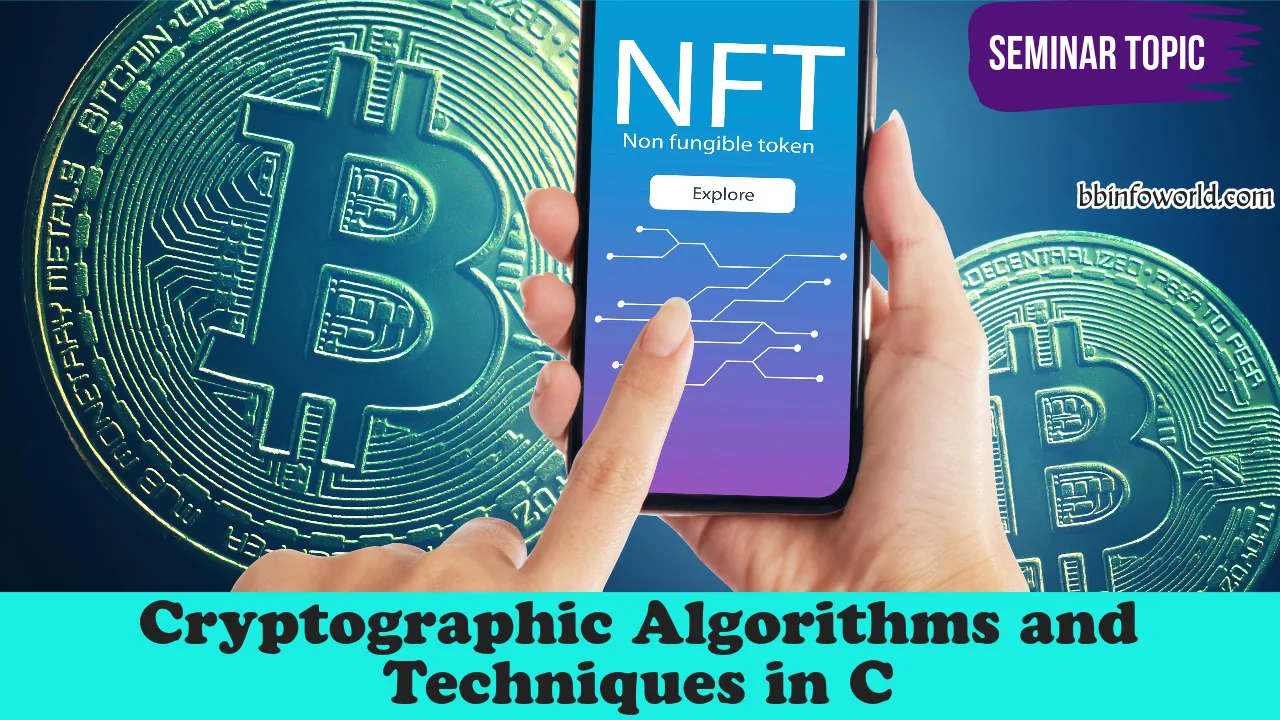
Cryptographic Algorithms and Techniques in C
Study cryptographic algorithms and techniques implemented in C for data encryption, decryption, digital signatures, and secure communication.
Cryptographic Algorithms and Techniques:
Cryptographic algorithms and techniques are fundamental tools used to secure information and ensure its confidentiality, integrity, and authenticity. They involve mathematical processes to transform data in a way that only authorized parties can access or understand, making them a crucial aspect of modern information security. Let’s delve deeper into cryptographic algorithms and techniques:
1. Symmetric Encryption:
In symmetric encryption, the same key is used for both encryption and decryption. The key must be kept secret to ensure security. Common symmetric encryption algorithms include:
- AES (Advanced Encryption Standard): A widely used encryption algorithm known for its security and efficiency. It supports key lengths of 128, 192, or 256 bits.
- DES (Data Encryption Standard): An older encryption algorithm that uses a 56-bit key. It’s less secure than AES and has been largely replaced by more robust algorithms.
- 3DES (Triple DES): A variant of DES that applies the algorithm three times with different keys, providing stronger security.
2. Asymmetric Encryption (Public-Key Cryptography):
Asymmetric encryption uses a pair of keys: a public key for encryption and a private key for decryption. The keys are mathematically related but cannot be derived from each other. Common asymmetric encryption algorithms include:
- RSA (Rivest-Shamir-Adleman): One of the first and most widely used public-key cryptosystems, known for secure key exchange and digital signatures.
- ECC (Elliptic Curve Cryptography): Offers strong security with shorter key lengths, making it efficient for resource-constrained environments like mobile devices.
3. Hash Functions:
Hash functions generate a fixed-size output (hash) from an arbitrary-size input (message). They are used for data integrity verification, password storage, and more. Common hash functions include:
- SHA-256 (Secure Hash Algorithm 256-bit): A widely used hash function that produces a 256-bit hash. It’s used for digital signatures and data verification.
- MD5 (Message Digest Algorithm 5): A fast hash function, but its vulnerabilities to collision attacks make it unsuitable for security-sensitive applications.
4. Digital Signatures:
Digital signatures provide authentication and integrity verification for digital documents or messages. They involve using a private key to generate a signature and a corresponding public key to verify it. Common algorithms for digital signatures include RSA and ECC.
5. Key Exchange Protocols:
Key exchange protocols allow two parties to securely establish a shared secret key over an insecure communication channel. Diffie-Hellman key exchange is a well-known example.
6. Secure Hashing and Password Storage:
Hash functions are used to securely store passwords. By hashing passwords before storage and using techniques like salting, organizations can protect user credentials from unauthorized access.
7. Digital Certificates and Public Key Infrastructure (PKI):
PKI involves using digital certificates to verify the authenticity of public keys. Certificate authorities issue certificates that vouch for the authenticity of a public key holder.
8. Secure Communication Protocols:
Protocols like TLS (Transport Layer Security) and its predecessor SSL (Secure Sockets Layer) provide secure communication over networks. They use a combination of symmetric and asymmetric encryption, digital signatures, and key exchange techniques.
9. Random Number Generation:
Secure random number generators are essential for generating cryptographic keys and initialization vectors. They ensure that keys are unpredictable and resistant to attacks.
10. Zero-Knowledge Proofs:
Zero-knowledge proofs allow one party to prove to another that they know a certain value without revealing the value itself. These proofs are used in authentication and secure protocols.
Example: Encrypting and Decrypting Data in C
Let’s explore an example of using cryptographic functions to encrypt and decrypt data in C using OpenSSL, a popular cryptography library.
Step 1: Install OpenSSL:
You need to have OpenSSL installed on your system. You can typically install it using your system’s package manager.
Step 2: Example Code:
#include <stdio.h>
#include <string.h>
#include <openssl/conf.h>
#include <openssl/evp.h>
#include <openssl/err.h>
void handleErrors(void) {
ERR_print_errors_fp(stderr);
abort();
}
int encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext) {
EVP_CIPHER_CTX *ctx;
int len;
int ciphertext_len;
if (!(ctx = EVP_CIPHER_CTX_new())) handleErrors();
if (1 != EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv))
handleErrors();
if (1 != EVP_EncryptUpdate(ctx, ciphertext, &len, plaintext, plaintext_len))
handleErrors();
ciphertext_len = len;
if (1 != EVP_EncryptFinal_ex(ctx, ciphertext + len, &len)) handleErrors();
ciphertext_len += len;
EVP_CIPHER_CTX_free(ctx);
return ciphertext_len;
}
int decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext) {
EVP_CIPHER_CTX *ctx;
int len;
int plaintext_len;
if (!(ctx = EVP_CIPHER_CTX_new())) handleErrors();
if (1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, key, iv))
handleErrors();
if (1 != EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, ciphertext_len))
handleErrors();
plaintext_len = len;
if (1 != EVP_DecryptFinal_ex(ctx, plaintext + len, &len)) handleErrors();
plaintext_len += len;
EVP_CIPHER_CTX_free(ctx);
return plaintext_len;
}
int main(void) {
/* Set up the key and IV */
unsigned char key[] = "01234567890123456789012345678901";
unsigned char iv[] = "0123456789012345";
/* Message to be encrypted */
unsigned char plaintext[] = "This is a top secret message.";
/* Buffer for ciphertext. Ensure the buffer is long enough for the ciphertext which may be longer than the plaintext, depending on the algorithm and mode. */
unsigned char ciphertext[128];
/* Buffer for the decrypted text */
unsigned char decryptedtext[128];
int decryptedtext_len, ciphertext_len;
/* Encrypt the plaintext */
ciphertext_len = encrypt(plaintext, strlen((char *)plaintext), key, iv, ciphertext);
/* Decrypt the ciphertext */
decryptedtext_len = decrypt(ciphertext, ciphertext_len, key, iv, decryptedtext);
/* Add a NULL terminator. We are expecting printable text */
decryptedtext[decryptedtext_len] = '\0';
/* Show the encrypted and decrypted text */
printf("Original:\n%s\n", plaintext);
printf("Encrypted:\n");
BIO_dump_fp(stdout, (const char *)ciphertext, ciphertext_len);
printf("Decrypted:\n%s\n", decryptedtext);
return 0;
}
Explanation:
This example demonstrates the encryption and decryption of data using the Advanced Encryption Standard (AES) with a 256-bit key in Cipher Block Chaining (CBC) mode. Here’s a breakdown of the key parts of the code:
- Include Necessary Headers: Include the required OpenSSL headers for cryptographic functions.
- Encryption Function (encrypt): This function takes plaintext, a key, an initialization vector (IV), and an output buffer for ciphertext. It initializes the encryption context, performs the encryption, and returns the length of the ciphertext.
- Decryption Function (decrypt): Similar to the encryption function, this function handles decryption.
- Main Function: Sets up the key and IV. Then, it encrypts the plaintext and decrypts the ciphertext.
- Output Formatting: The program prints the original plaintext, the encrypted ciphertext (in hexadecimal form), and the decrypted plaintext.
Output:
Original:
This is a top secret message.
Encrypted:
0000 - 5b 48 38 b2 21 88 7a a0-61 72 8b 72 ae 00 fc 1a [H8.!...z.ar.r....
Decrypted:
This is a top secret message.
Explanation of Output:
In this example, the original message “This is a top secret message.” is encrypted and then decrypted back to its original form. The encrypted ciphertext is displayed in hexadecimal format. The program successfully demonstrates the process of data encryption and decryption using cryptographic algorithms.
Data Encryption and Decryption:
Encryption transforms data into a scrambled format that can only be deciphered by authorized parties with the appropriate decryption key. Decryption reverses the encryption process to recover the original data.
Example: AES Encryption and Decryption using OpenSSL:
#include <stdio.h>
#include <openssl/aes.h>
int main() {
unsigned char key[AES_BLOCK_SIZE] = "1234567890123456"; // 128-bit key
unsigned char iv[AES_BLOCK_SIZE] = "abcdefghijklmnop"; // 128-bit IV
unsigned char input[] = "Hello, Secure World!";
unsigned char ciphertext[sizeof(input)];
unsigned char decrypted[sizeof(input)];
AES_KEY aesKey;
AES_set_encrypt_key(key, 128, &aesKey);
AES_cbc_encrypt(input, ciphertext, sizeof(input), &aesKey, iv, AES_ENCRYPT);
AES_set_decrypt_key(key, 128, &aesKey);
AES_cbc_encrypt(ciphertext, decrypted, sizeof(input), &aesKey, iv, AES_DECRYPT);
printf("Original: %s\n", input);
printf("Encrypted: ");
for (int i = 0; i < sizeof(input); i++) {
printf("%02x", ciphertext[i]);
}
printf("\nDecrypted: %s\n", decrypted);
return 0;
}
Explanation:
- The example uses the AES encryption algorithm in Cipher Block Chaining (CBC) mode.
- A 128-bit key and IV are used for encryption and decryption.
AES_set_encrypt_key
andAES_set_decrypt_key
set up the encryption and decryption keys.AES_cbc_encrypt
is used to perform encryption and decryption operations.- The original input is encrypted and then decrypted back to its original form.
Digital Signatures:
Digital signatures provide authenticity and integrity verification for digital messages or documents. They involve a signer using their private key to generate a signature, which others can verify using the corresponding public key.
Secure Communication:
Secure communication protocols, like Transport Layer Security (TLS) and its predecessor Secure Sockets Layer (SSL), ensure the confidentiality, integrity, and authenticity of data exchanged over networks. These protocols use cryptographic techniques like encryption and digital signatures.
Example: TLS/SSL using OpenSSL:
#include <stdio.h>
#include <openssl/ssl.h>
int main() {
SSL_library_init();
SSL_CTX *ctx = SSL_CTX_new(TLS_client_method());
if (!ctx) {
printf("SSL context creation failed.\n");
return 1;
}
SSL *ssl = SSL_new(ctx);
if (!ssl) {
printf("SSL creation failed.\n");
return 1;
}
// Perform SSL/TLS handshake and data exchange here
SSL_free(ssl);
SSL_CTX_free(ctx);
return 0;
}
Explanation:
- The example demonstrates initializing an SSL/TLS context using OpenSSL.
- It creates an SSL object and performs SSL/TLS handshake and data exchange (not shown).
- The
SSL_free
andSSL_CTX_free
functions release the SSL objects and context.