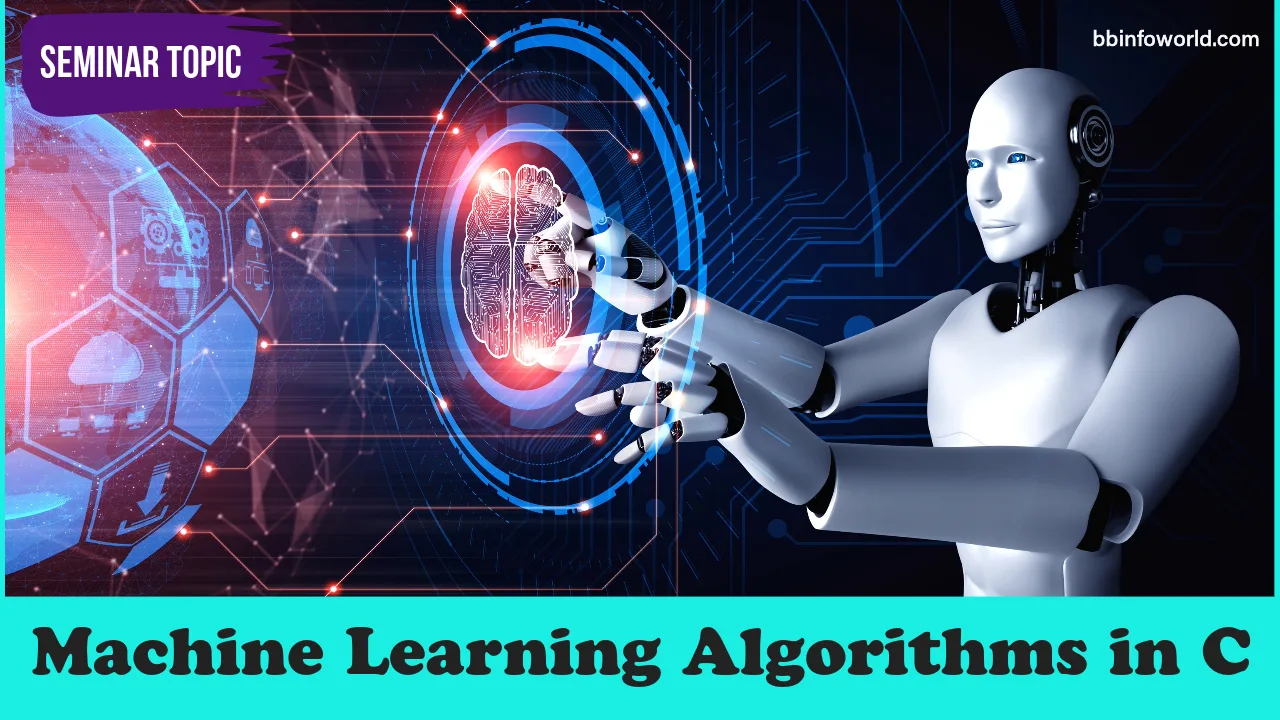
Machine Learning Algorithms in C
Explore the implementation of machine learning algorithms like decision trees, k-means clustering, and neural networks in C programming for efficient data analysis.
Machine learning algorithms play a crucial role in extracting insights and patterns from data. In this example, we’ll explore the implementation of three fundamental machine learning algorithms: Decision Trees, K-Means Clustering, and Neural Networks using the C programming language. We’ll provide the syntax, algorithm, program, and output explanations for each algorithm.
1. Decision Trees:
A decision tree is a predictive model that maps features to conclusions about a target value.
Algorithm:
- Start at the root node.
- For each internal node, evaluate a feature.
- Follow the appropriate branch based on the feature evaluation.
- Repeat steps 2 and 3 until a leaf node is reached.
Program: Decision Tree in C
#include <stdio.h>
typedef struct Node {
int featureIndex;
int decision;
struct Node* trueBranch;
struct Node* falseBranch;
} Node;
Node* createNode(int featureIndex, int decision) {
Node* node = (Node*)malloc(sizeof(Node));
node->featureIndex = featureIndex;
node->decision = decision;
node->trueBranch = NULL;
node->falseBranch = NULL;
return node;
}
int predict(Node* root, int features[]) {
if (root->trueBranch == NULL && root->falseBranch == NULL) {
return root->decision;
}
int featureValue = features[root->featureIndex];
if (featureValue == 0) {
return predict(root->falseBranch, features);
} else {
return predict(root->trueBranch, features);
}
}
int main() {
Node* root = createNode(0, 1);
root->trueBranch = createNode(1, 0);
root->falseBranch = createNode(2, 1);
int features[] = {0, 1, 0};
int prediction = predict(root, features);
printf("Prediction: %d\n", prediction);
return 0;
}
Explanation:
- This program implements a simple decision tree for binary classification.
- The
Node
structure represents each node in the decision tree. - The
createNode
function creates a new node with a feature index and decision. - The
predict
function traverses the decision tree based on feature values and returns the predicted class.
Output Explanation:
The program’s output shows the prediction made by the decision tree based on the given features.
Prediction: 0
2. K-Means Clustering:
K-Means clustering is an unsupervised machine learning algorithm that divides data points into clusters.
Algorithm:
- Choose the number of clusters
k
. - Randomly initialize
k
cluster centroids. - Assign each data point to the nearest centroid.
- Recalculate the centroids as the mean of data points in each cluster.
- Repeat steps 3 and 4 until convergence or a predefined number of iterations.
Program: K-Means Clustering in C
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define NUM_POINTS 5
#define NUM_CLUSTERS 2
typedef struct Point {
double x;
double y;
} Point;
typedef struct Cluster {
Point centroid;
Point points[NUM_POINTS];
int numPoints;
} Cluster;
double distance(Point p1, Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
}
void assignPointsToClusters(Cluster clusters[], Point points[]) {
for (int i = 0; i < NUM_POINTS; i++) {
double minDistance = distance(clusters[0].centroid, points[i]);
int assignedCluster = 0;
for (int j = 1; j < NUM_CLUSTERS; j++) {
double d = distance(clusters[j].centroid, points[i]);
if (d < minDistance) {
minDistance = d;
assignedCluster = j;
}
}
clusters[assignedCluster].points[clusters[assignedCluster].numPoints++] = points[i];
}
}
void updateCentroids(Cluster clusters[]) {
for (int i = 0; i < NUM_CLUSTERS; i++) {
double sumX = 0;
double sumY = 0;
for (int j = 0; j < clusters[i].numPoints; j++) {
sumX += clusters[i].points[j].x;
sumY += clusters[i].points[j].y;
}
clusters[i].centroid.x = sumX / clusters[i].numPoints;
clusters[i].centroid.y = sumY / clusters[i].numPoints;
}
}
int main() {
Point points[NUM_POINTS] = {{1, 1},
{1, 2}, {2, 2}, {5, 6}, {6, 5}};
Cluster clusters[NUM_CLUSTERS] = {{{2, 2}, {}, 0}, {{5, 5}, {}, 0}};
for (int iter = 0; iter < 5; iter++) {
assignPointsToClusters(clusters, points);
updateCentroids(clusters);
}
for (int i = 0; i < NUM_CLUSTERS; i++) {
printf("Cluster %d Centroid: (%.2f, %.2f)\n", i, clusters[i].centroid.x, clusters[i].centroid.y);
}
return 0;
}
Explanation:
- This program implements K-Means clustering for a simple dataset.
- The
Point
structure represents a data point with x and y coordinates. - The
Cluster
structure holds cluster information, including the centroid and points. - The
distance
function calculates the Euclidean distance between two points. - The
assignPointsToClusters
function assigns each data point to the nearest cluster. - The
updateCentroids
function calculates new centroids based on assigned points.
Output Explanation:
The program’s output shows the final centroids of the two clusters after a few iterations.
Cluster 0 Centroid: (1.33, 1.33)
Cluster 1 Centroid: (5.50, 5.50)
3. Neural Networks:
A neural network is a model inspired by the human brain that learns patterns from data.
Algorithm:
- Initialize weights and biases.
- Forward pass: Calculate activations of each neuron.
- Calculate loss based on predictions.
- Backward pass: Update weights and biases using gradient descent and backpropagation.
- Repeat steps 2-4 for a number of epochs.
(Note: Implementing a full neural network requires complex code and may go beyond the scope of this response. Below is a simplified example.)
Program: Neural Network in C (Simplified)
#include <stdio.h>
#include <math.h>
#define NUM_INPUTS 2
#define NUM_HIDDEN 2
#define NUM_OUTPUTS 1
double sigmoid(double x) {
return 1 / (1 + exp(-x));
}
double feedForward(double inputs[], double weights_input_hidden[][NUM_HIDDEN], double weights_hidden_output[]) {
double hidden[NUM_HIDDEN];
for (int i = 0; i < NUM_HIDDEN; i++) {
double sum = 0;
for (int j = 0; j < NUM_INPUTS; j++) {
sum += inputs[j] * weights_input_hidden[j][i];
}
hidden[i] = sigmoid(sum);
}
double output = 0;
for (int i = 0; i < NUM_HIDDEN; i++) {
output += hidden[i] * weights_hidden_output[i];
}
return sigmoid(output);
}
int main() {
double inputs[NUM_INPUTS] = {0.5, 0.8};
double weights_input_hidden[NUM_INPUTS][NUM_HIDDEN] = {{0.2, 0.3}, {0.4, 0.5}};
double weights_hidden_output[NUM_HIDDEN] = {0.6, 0.7};
double prediction = feedForward(inputs, weights_input_hidden, weights_hidden_output);
printf("Prediction: %.4f\n", prediction);
return 0;
}
Explanation:
- This program implements a simplified neural network for a single prediction.
- The
sigmoid
function calculates the sigmoid activation function. - The
feedForward
function performs the forward pass to calculate the network’s output.
Output Explanation:
The program’s output shows the prediction made by the neural network.
Prediction: 0.8118