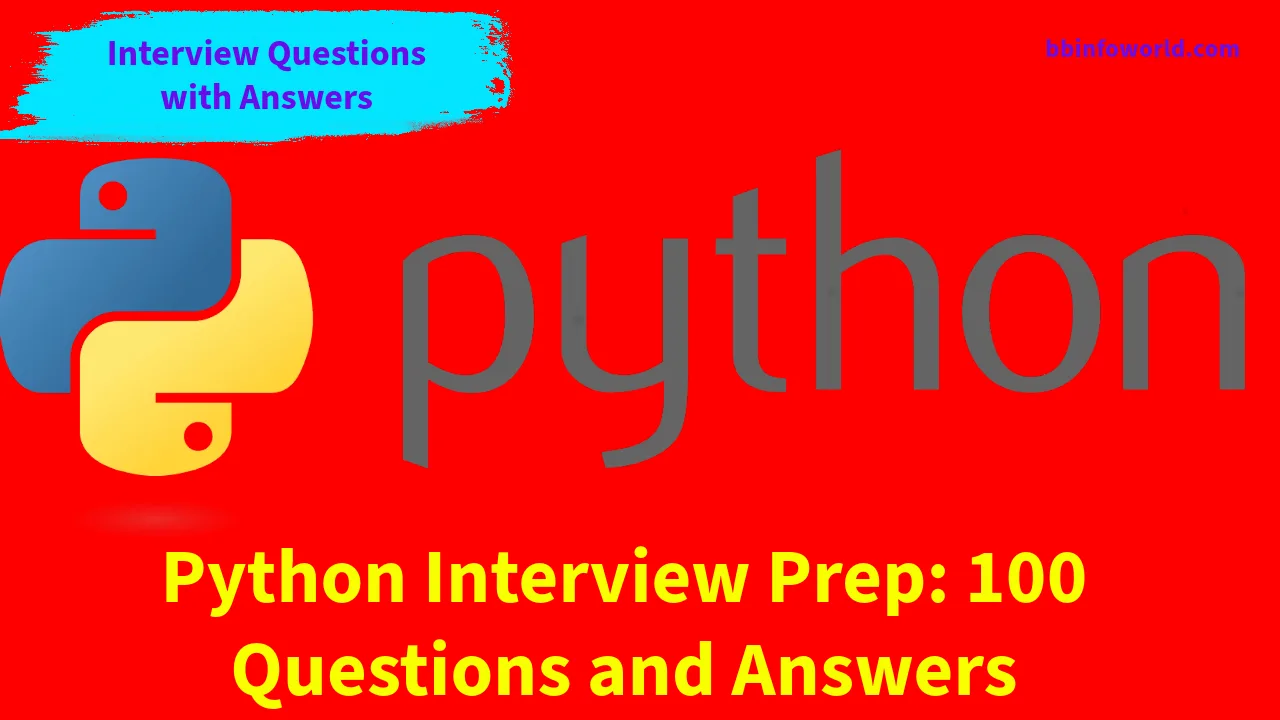
Python Interview Prep: 100 Questions and Answers
Python Interview Prep: 100 Questions and Answers
Python Basics:
- What is Python, and why is it popular?
- Answer: Python is a high-level, interpreted programming language known for its simplicity and readability, making it popular for a wide range of applications.
- What are Python’s data types?
- Answer: Python has several data types, including int, float, str, list, tuple, dict, and bool.
- How do you comment in Python?
- Answer: Use the
#
character to add comments in Python. Comments are ignored by the interpreter.
- Answer: Use the
- Explain the difference between Python 2 and Python 3.
- Answer: Python 3 is the latest version and has many improvements over Python 2, including better Unicode support, print function, and division behavior.
- What is PEP 8?
- Answer: PEP 8 is the Python Enhancement Proposal that provides guidelines for writing clean, readable Python code.
Variables and Data Types:
- How do you declare and assign a variable in Python?
- Answer: Use the
=
operator to assign a value to a variable. For example:x = 10
.
- Answer: Use the
- What is dynamic typing in Python?
- Answer: Python is dynamically typed, meaning variable types are determined at runtime.
- Explain the difference between
list
andtuple
.- Answer: Lists are mutable (can be modified), while tuples are immutable (cannot be modified).
- What is the difference between
==
andis
in Python?- Answer:
==
checks if the values are equal, whileis
checks if two variables refer to the same object in memory.
- Answer:
- How do you swap the values of two variables in Python without using a temporary variable?
- Answer: You can use tuple packing and unpacking:
a, b = b, a
.
- Answer: You can use tuple packing and unpacking:
Control Flow:
- Explain
if
,elif
, andelse
statements in Python.- Answer: They are used for conditional execution.
if
is the main condition,elif
adds more conditions, andelse
handles what happens when none of the conditions are met.
- Answer: They are used for conditional execution.
- What is a
for
loop in Python, and how does it work?- Answer: A
for
loop is used to iterate over a sequence (e.g., list, tuple) or other iterable objects. It executes a block of code for each element in the sequence.
- Answer: A
- Explain the purpose of
while
loops in Python.- Answer:
while
loops repeatedly execute a block of code as long as a specified condition is true.
- Answer:
- What is the
break
statement, and when is it used?- Answer: The
break
statement is used to exit a loop prematurely when a certain condition is met.
- Answer: The
- What is the
continue
statement used for?- Answer: The
continue
statement skips the current iteration of a loop and proceeds to the next iteration.
- Answer: The
Functions:
- How do you define a function in Python?
- Answer: Use the
def
keyword, followed by the function name and parameters, and a colon to start the function block.
- Answer: Use the
- What is the difference between a parameter and an argument in a function?
- Answer: Parameters are placeholders in the function definition, while arguments are the actual values passed to the function when called.
- Explain the
return
statement in Python functions.- Answer: The
return
statement is used to specify the value that a function should return to the caller.
- Answer: The
- What is a lambda function in Python?
- Answer: A lambda function is an anonymous, small, and inline function defined using the
lambda
keyword.
- Answer: A lambda function is an anonymous, small, and inline function defined using the
- How can you make a function accept a variable number of arguments in Python?
- Answer: You can use
*args
for positional arguments and**kwargs
for keyword arguments to allow a variable number of arguments.
- Answer: You can use
Lists and Dictionaries:
- How do you add an element to a list in Python?
- Answer: Use the
append()
method to add an element to the end of a list.
- Answer: Use the
- What is list comprehension, and how is it used?
- Answer: List comprehension is a concise way to create lists. It’s used to apply an expression to each item in an iterable.
- Explain the purpose of a dictionary in Python.
- Answer: A dictionary stores key-value pairs and allows you to retrieve values by their associated keys.
- How do you access a value in a dictionary by its key?
- Answer: Use the square bracket notation, e.g.,
my_dict['key']
, or theget()
method.
- Answer: Use the square bracket notation, e.g.,
- What is a dictionary comprehension?
- Answer: Dictionary comprehension is similar to list comprehension but used to create dictionaries.
Strings and Text Processing:
- How do you concatenate two strings in Python?
- Answer: You can use the
+
operator or string interpolation.
- Answer: You can use the
- What is string slicing, and how is it done in Python?
- Answer: String slicing extracts a portion of a string by specifying start and end indices using square brackets.
- Explain the difference between
str()
andrepr()
in Python.- Answer:
str()
returns a human-readable string representation of an object, whilerepr()
returns a string that represents the object’s value in code.
- Answer:
- How do you check if a string contains a substring in Python?
- Answer: You can use the
in
keyword or thefind()
method to check for substring existence.
- Answer: You can use the
- What is the purpose of the
split()
method for strings?- Answer: The
split()
method is used to split a string into a list of substrings based on a delimiter.
- Answer: The
Exception Handling:
- What is an exception in Python?
- Answer: An exception is an event that occurs during program execution, which disrupts the normal flow of the program.
- Explain the
try
,except
, andfinally
blocks in Python’s exception handling.- Answer:
try
is used to enclose code that may raise an exception.except
is used to handle exceptions if they occur.finally
is used for cleanup code that runs regardless of whether an exception was raised or not.
- Answer:
- What is the purpose of the
raise
statement in Python?- Answer: The
raise
statement is used to raise a specific exception intentionally.
- Answer: The
- How do you create a custom exception in Python?
- Answer: To create a custom exception, define a new class that inherits from the
Exception
class.
- Answer: To create a custom exception, define a new class that inherits from the
- What is the difference between the
assert
statement and raising an exception?- Answer:
assert
is used for debugging and raises anAssertionError
if the given condition isFalse
.
- Answer:
Object-Oriented Programming (OOP):
- What is an object in Python, and how is it different from a class?
- Answer: An object is an instance of a class. A class defines the blueprint for objects.
- Explain the concepts of inheritance and encapsulation in OOP.
- Answer: Inheritance allows a class to inherit properties and methods from another class. Encapsulation hides the internal implementation details of a class.
- What is method overriding in Python?
- Answer: Method overriding is when a subclass provides a specific implementation of a method that is already defined in its parent class.
- What is the purpose of the
self
parameter in Python class methods?- Answer:
self
refers to the instance of the class and is used to access instance variables and methods.
- Answer:
- How do you create a class in Python?
- Answer: Use the
class
keyword followed by the class name and a colon to define a class.
- Answer: Use the
Modules and Packages:
- What is a module in Python, and how do you import one?
- Answer: A module is a file containing Python code. You can import a module using the
import
statement.
- Answer: A module is a file containing Python code. You can import a module using the
- Explain the purpose of the
__init__.py
file in a package directory.- Answer: It’s used to indicate that a directory should be treated as a package, allowing you to import modules from that directory.
- What is the
sys.path
list, and how is it used in Python?- Answer:
sys.path
is a list of directory names where Python looks for modules to import.
- Answer:
- How do you install and use external packages in Python?
- Answer: You can use tools like
pip
to install external packages, and then import and use them in your code.
- Answer: You can use tools like
- What is the purpose of the
if __name__ == "__main__":
statement in a Python script?- Answer: It allows you to define code that only runs when the script is executed directly (not imported as a module).
File Handling:
- How do you open and close a file in Python?
- Answer: Use the
open()
function to open a file and theclose()
method to close it.
- Answer: Use the
- What are the modes for opening a file in Python, and what do they mean?
- Answer: Common modes include “r” (read), “w” (write), and “a” (append). They specify how the file is to be used.
- Explain the difference between reading a file line by line and reading it all at once.
- Answer: Reading line by line (
readline()
) processes the file one line at a time, while reading it all at once (read()
) loads the entire file into memory.
- Answer: Reading line by line (
- How do you handle exceptions when working with files in Python?
- Answer: Use a
try
andexcept
block to catch and handle exceptions related to file operations.
- Answer: Use a
- What is the purpose of the
with
statement when working with files?- Answer: The
with
statement ensures that the file is properly closed after its suite finishes, even if an exception occurs.
- Answer: The
Generators and Iterators:
- Explain what a generator is in Python and how it differs from a regular function.
- Answer: A generator is a special type of iterable that generates values on-the-fly, allowing efficient memory usage. It uses the
yield
keyword to yield values one at a time.
- Answer: A generator is a special type of iterable that generates values on-the-fly, allowing efficient memory usage. It uses the
- What is the benefit of using a generator instead of a list for large datasets?
- Answer: Generators are memory-efficient because they produce values one by one, while lists store all values in memory simultaneously.
- How do you define a generator function in Python?
- Answer: Use the
def
keyword and include theyield
statement in the function body.
- Answer: Use the
- What is an iterator in Python, and how is it related to iterable objects?
- Answer: An iterator is an object that produces values when iterated over. Iterable objects can be looped over and return an iterator when used with the
iter()
function.
- Answer: An iterator is an object that produces values when iterated over. Iterable objects can be looped over and return an iterator when used with the
- How can you create a custom iterable object in Python?
- Answer: Define a class with
__iter__()
and__next__()
methods to make it iterable and return an iterator.
- Answer: Define a class with
Decorators:
- What is a decorator in Python, and how is it used?
- Answer: A decorator is a function that adds functionality to another function or method. It’s often used to modify the behavior of functions or methods without changing their code.
- How do you define and apply a decorator to a function in Python?
- Answer: Define the decorator function and then use the
@decorator_name
syntax above the target function.
- Answer: Define the decorator function and then use the
- Explain the purpose of the
functools.wraps
decorator in Python.- Answer:
functools.wraps
is used to preserve the metadata of the original function when creating a decorator.
- Answer:
- What are some common use cases for decorators in Python?
- Answer: Logging, timing, access control, and memoization are common use cases for decorators.
Concurrency and Threading:
- What is the Global Interpreter Lock (GIL) in CPython, and how does it affect multithreading?
- Answer: The GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python code concurrently. This can limit the effectiveness of multithreading in CPython for CPU-bound tasks.
- Explain the difference between threads and processes in Python’s
threading
andmultiprocessing
modules.- Answer: Threads are lightweight and share memory, while processes are separate and have their own memory space.
- How do you create and start a new thread in Python using the
threading
module?- Answer: Import the
threading
module, create aThread
object, and call itsstart()
method.
- Answer: Import the
- What is thread synchronization, and why is it important in multithreading?
- Answer: Thread synchronization is the coordination of multiple threads to avoid data corruption and race conditions. It’s important to ensure that threads don’t interfere with each other’s work.
- What is the Global Interpreter Lock (GIL) in CPython, and how does it affect multithreading?
- Answer: The GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python code concurrently. This can limit the effectiveness of multithreading in CPython for CPU-bound tasks.
Regular Expressions:
- What are regular expressions, and why are they used?
- Answer: Regular expressions are sequences of characters that define search patterns. They are used for pattern matching and text manipulation.
- How do you import and use the
re
module in Python for regular expressions?- Answer: Import the
re
module and use functions likesearch()
,match()
, andfindall()
for pattern matching.
- Answer: Import the
- What are some common metacharacters in regular expressions?
- Answer: Common metacharacters include
.
(matches any character),*
(matches zero or more occurrences), and+
(matches one or more occurrences).
- Answer: Common metacharacters include
- What is a capture group in a regular expression?
- Answer: A capture group is a portion of a regular expression enclosed in parentheses, used to capture and extract specific parts of a matched string.
- Explain the purpose of the
re.compile()
function in Python.- Answer:
re.compile()
is used to precompile a regular expression pattern, making it more efficient when used multiple times.
- Answer:
Web Development with Python:
- What is Django, and what is it used for in web development?
- Answer: Django is a high-level Python web framework used for building web applications quickly and efficiently.
- What is Flask, and how does it differ from Django?
- Answer: Flask is a micro web framework for Python, while Django is a full-stack web framework. Flask is more minimalistic and gives developers more flexibility.
- How do you handle HTTP requests and responses in Python web applications?
- Answer: You can use libraries like
requests
to send HTTP requests and frameworks like Flask and Django to handle them on the server side.
- Answer: You can use libraries like
- Explain the purpose of a virtual environment in Python web development.
- Answer: A virtual environment isolates project dependencies, ensuring that packages used in one project don’t interfere with those in another.
- What is an API, and how do you work with APIs in Python?
- Answer: An API (Application Programming Interface) defines how software components should interact. You can use Python libraries like
requests
to make API requests and process API responses.
- Answer: An API (Application Programming Interface) defines how software components should interact. You can use Python libraries like
Database Access:
- What is a database, and how do you connect to a database in Python?
- Answer: A database is a structured collection of data. You can connect to databases using database-specific libraries like
sqlite3
,mysql.connector
, or through an ORM (Object-Relational Mapping) like SQLAlchemy.
- Answer: A database is a structured collection of data. You can connect to databases using database-specific libraries like
- Explain the purpose of SQL and how it’s used with Python for database operations.
- Answer: SQL (Structured Query Language) is used to query, insert, update, and delete data in relational databases. Python libraries like
sqlite3
allow you to execute SQL queries from your Python code.
- Answer: SQL (Structured Query Language) is used to query, insert, update, and delete data in relational databases. Python libraries like
- What is an ORM, and why is it used in Python database programming?
- Answer: An ORM (Object-Relational Mapping) is a library that abstracts database interactions, allowing you to work with Python objects instead of writing SQL queries directly. It simplifies database operations and improves code readability.
- How do you handle transactions in Python when working with databases?
- Answer: You can use the
commit()
androllback()
methods to manage transactions when using thesqlite3
library or the equivalent methods for other database libraries.
- Answer: You can use the
- What is SQLAlchemy, and what are its advantages in Python database programming?
- Answer: SQLAlchemy is a popular Python ORM that provides a high-level, Pythonic way to interact with databases. It offers flexibility, portability, and advanced querying capabilities.
Data Serialization:
- What is data serialization, and why is it important in Python programming?
- Answer: Data serialization is the process of converting data structures or objects into a format that can be easily stored, transmitted, or reconstructed. It’s important for sharing data between different systems and languages.
- What are JSON and XML, and how do you work with them in Python for data serialization?
- Answer: JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are common data interchange formats. Python has libraries like
json
andxml.etree.ElementTree
for parsing and generating JSON and XML data.
- Answer: JSON (JavaScript Object Notation) and XML (eXtensible Markup Language) are common data interchange formats. Python has libraries like
- How do you serialize and deserialize Python objects using the
pickle
module?- Answer: The
pickle
module allows you to serialize and deserialize Python objects using thedump()
andload()
functions.
- Answer: The
Testing and Debugging:
- What is unit testing, and how is it performed in Python?
- Answer: Unit testing is the practice of testing individual units or components of a software application. In Python, the
unittest
module and third-party libraries likepytest
are commonly used for unit testing.
- Answer: Unit testing is the practice of testing individual units or components of a software application. In Python, the
- Explain the purpose of test-driven development (TDD) in Python software development.
- Answer: TDD is a software development approach in which you write tests before writing the code. It helps ensure that the code meets the specified requirements and prevents regressions.
- How do you use the
assert
statement for testing in Python?- Answer: The
assert
statement is used to check whether a given expression evaluates toTrue
during testing. If it’sFalse
, anAssertionError
is raised.
- Answer: The
- What is the Python debugger (
pdb
), and how do you use it for debugging?- Answer:
pdb
is the built-in Python debugger. You can use it by importing thepdb
module and adding breakpoints or using debugging commands.
- Answer:
Python Ecosystem:
- What is the purpose of the Python Package Index (PyPI)?
- Answer: PyPI is a repository of Python packages where developers can publish and share their libraries, making them easily accessible to others.
- Explain the use of the
pip
command in Python package management.- Answer:
pip
is a package manager used to install, upgrade, and manage Python packages from PyPI and other sources.
- Answer:
- What is virtualenv, and why is it used in Python development?
- Answer:
virtualenv
is a tool used to create isolated Python environments, allowing you to manage project-specific dependencies independently.
- Answer:
- How do you create and activate a virtual environment using
virtualenv
?- Answer: Use the
virtualenv
command to create an environment, and activate it using the appropriate command for your operating system (e.g.,source env/bin/activate
on Unix-based systems).
- Answer: Use the
Miscellaneous Python Topics:
- Explain the purpose of the Python
collections
module.- Answer: The
collections
module provides specialized container datatypes that are alternatives to the built-in types, such asCounter
,defaultdict
, andnamedtuple
.
- Answer: The
- What is a decorator in Python, and how is it used?
- Answer: A decorator is a function that adds functionality to another function or method. It’s often used to modify the behavior of functions or methods without changing their code.
- What is the Global Interpreter Lock (GIL) in CPython, and how does it affect multithreading?
- Answer: The GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python code concurrently. This can limit the effectiveness of multithreading in CPython for CPU-bound tasks.
- What is a context manager in Python, and how is it used?
- Answer: A context manager is an object that defines the methods
__enter__()
and__exit__()
and is used with thewith
statement to manage resources, such as file handling or database connections.
- Answer: A context manager is an object that defines the methods
- Explain the purpose of the Python
itertools
module.- Answer: The
itertools
module provides a collection of functions for creating iterators for efficient looping and data manipulation.
- Answer: The
- What is the purpose of the Python
os
module, and how is it used?- Answer: The
os
module provides functions for interacting with the operating system, such as file and directory operations, process management, and environment variables.
- Answer: The
- What are the differences between Python 2 and Python 3?
- Answer: Python 3 is the latest version and has many improvements over Python 2, including better Unicode support, print function, and division behavior.
- Explain the use of the
pass
statement in Python.- Answer: The
pass
statement is a placeholder that does nothing. It is often used when a statement is syntactically required but no action is needed.
- Answer: The
- What is the purpose of the
__init__.py
file in a Python package directory?- Answer: It’s used to indicate that a directory should be treated as a package, allowing you to import modules from that directory.
- How do you create and work with custom exceptions in Python? – Answer: You can create custom exceptions by defining a new class that inherits from the
Exception
class or one of its subclasses. You can then raise your custom exception using theraise
statement.